C++ Exercises: Create a new string of length 2, using first two characters of a given string
Two Characters or Pad with #
Write a C++ program to create a new string of length 2, using the first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that manipulates the input string based on its length
string test(string s1)
{
// Check if the length of the string is greater than or equal to 2
if (s1.length() >= 2)
{
s1 = s1.substr(0, 2); // Take the first two characters of s1
}
// If the length of the string is greater than 0 and less than 2
else if (s1.length() > 0)
{
s1 = s1.substr(0, 1) + "#"; // Take the first character of s1 and append '#' to it
}
else // If the string is empty
{
s1 = "##"; // Assign "##" to s1
}
return s1; // Return the modified string
}
// Main function
int main()
{
cout << test("Hello") << endl; // Output the result of test function with "Hello"
cout << test("Python") << endl; // Output the result of test function with "Python"
cout << test("a") << endl; // Output the result of test function with "a"
cout << test("") << endl; // Output the result of test function with an empty string
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
He Py a# ##
Visual Presentation:
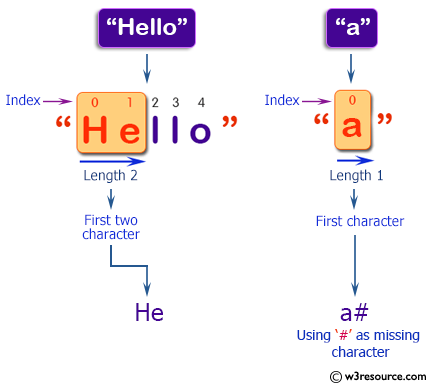
Flowchart:
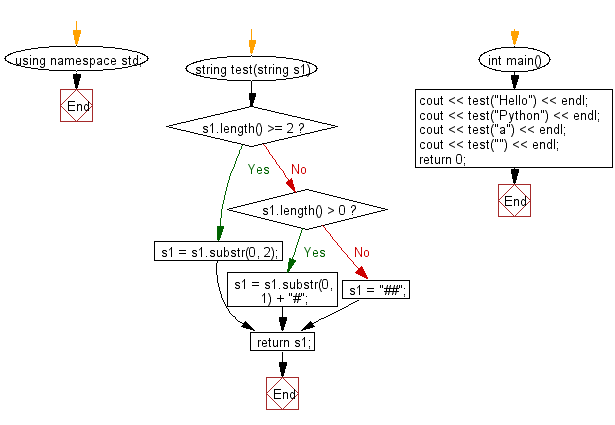
For more Practice: Solve these Related Problems:
- Write a C++ program that extracts the first two characters of a string and, if the string is too short, appends '#' until the result has length 2.
- Write a C++ program to create a new string of length 2 by taking the first two characters of the input; if missing, fill the gap with the '#' character using conditional operators.
- Write a C++ program that reads a string and outputs its first two characters; if the string has one or zero characters, pad the output on the right with '#' to achieve two characters.
- Write a C++ program to process a string so that the output is always two characters long by extracting or padding with '#' as needed.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string of length 2 starting at the given index of a given string.
Next: Write a C++ program to create a new string taking the first character from a given string and the last character from another given string. If the length of any given string is 0, use '#' as its missing character.
What is the difficulty level of this exercise?