C++ Exercises: Create a new string taking 3 characters from the middle of a given string at least 3
Middle Characters String
Write a C++ program that takes at least 3 characters from the middle of a given string that would be used to create a string.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that extracts a substring of length 2 from the given index in the input string
string test(string s1, int index)
{
// Conditional operator to check if index+2 is within the length of the string
return index + 2 <= s1.length() ? s1.substr(index, 2) : s1.substr(0, 2);
// Return a substring starting from the given index of length 2 if possible, otherwise return the first two characters of s1
}
// Main function
int main()
{
cout << test("Hello", 1) << endl; // Output the result of test function with "Hello" and index=1
cout << test("Python", 2) << endl; // Output the result of test function with "Python" and index=2
cout << test("on", 1) << endl; // Output the result of test function with "on" and index=1
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
el th on
Visual Presentation:
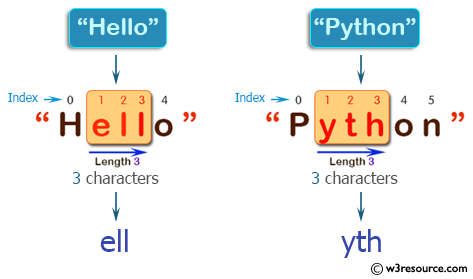
Flowchart:
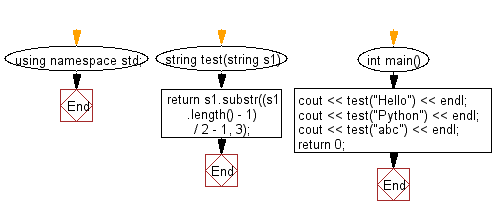
For more Practice: Solve these Related Problems:
- Write a C++ program to extract a substring from the middle of a string, using the middle third of its characters if the length is odd or even.
- Write a C++ program that reads a string and prints a new string consisting of characters from the center, excluding the extreme ends.
- Write a C++ program to determine and output the central characters of an input string, using calculations to find the midpoint.
- Write a C++ program that accepts a string and extracts a segment from its middle portion, ensuring a balanced substring is returned.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string of length 2 starting at the given index of a given string.
Next: Write a C++ program to create a new string of length 2, using first two characters of a given string. If the given string length is less than 2 use '#' as missing characters.
What is the difficulty level of this exercise?