C++ Exercises: Create a new string using the first and last n characters from a given string of length at least n
First and Last n Characters from String
Write a C++ program to create a new string using the first and last n characters from a given string of length at least n.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that concatenates substrings based on the given indices
string test(string s1, int n)
{
return s1.substr(0, n) + s1.substr(s1.length() - n); // Concatenate a substring from index 0 to n and a substring from length-n to the end of s1
}
// Main function
int main()
{
cout << test("Hello", 1) << endl; // Output the result of test function with "Hello" and n=1
cout << test("Python", 2) << endl; // Output the result of test function with "Python" and n=2
cout << test("on", 1) << endl; // Output the result of test function with "on" and n=1
cout << test("o", 1) << endl; // Output the result of test function with "o" and n=1
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
Ho Pyon on oo
Visual Presentation:
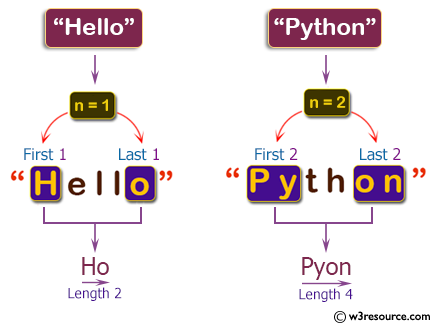
Flowchart:
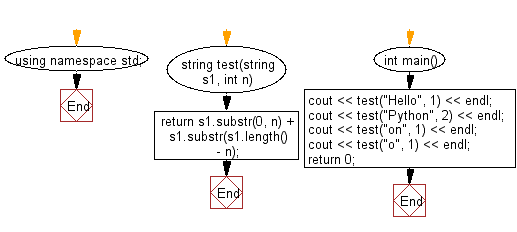
For more Practice: Solve these Related Problems:
- Write a C++ program to read a string and an integer n, then output a new string made by concatenating the first n and last n characters of the input.
- Write a C++ program that extracts the first and last n characters from a given string and joins them into a single output string.
- Write a C++ program to combine the first n and last n letters of an input string and display the resultant string.
- Write a C++ program that accepts a string and a number n, then prints the concatenation of its leading and trailing n characters.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string using the two middle characters of a given string of even length (at least 2).
Next: Write a C++ program to create a new string of length 2 starting at the given index of a given string.
What is the difficulty level of this exercise?