C++ Exercises: Create a new string using the two middle characters of a given string of even length
Two Middle Characters of Even-Length String
Write a C++ program to create a string using the two middle characters of a given string of even length (at least 2).
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function that extracts a substring of length 2 from the middle of the input string
string test(string s1)
{
return s1.substr(s1.length() / 2 - 1, 2); // Extracts a substring of length 2 starting from the middle (or slightly left of the middle) of the input string
}
// Main function
int main()
{
cout << test("Hell") << endl; // Output the result of test function with "Hell"
cout << test("JS") << endl; // Output the result of test function with "JS"
return 0; // Return statement indicating successful termination of the program
}
Sample Output:
el JS
Visual Presentation:
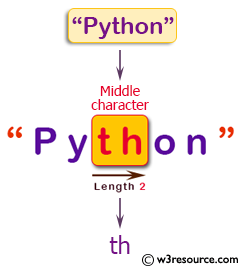
Flowchart:
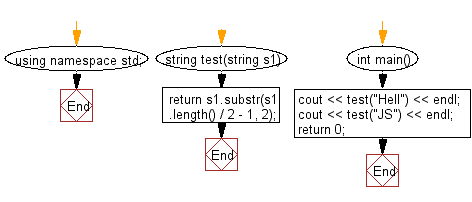
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string without the first and last character of a given string of any length.
Next: Write a C++ program to create a new string using the first and last n characters from a given string of length at least n.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics