C++ Exercises: Create a new string without the first and last character of a given string of length atleast two
Remove First and Last Characters
Write a C++ program to create a new string without the first and last characters of a given string of length at least two.
Sample Solution:
C++ Code :
#include <iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function definition to process the input string and return a substring
string test(string s1) // Function named 'test' taking a string parameter 's1'
{
return s1.substr(1) // Extract a substring from index 1 to the end of the string 's1'
.substr(0, s1.length() - 2); // Further, extract a substring from index 0 up to the length of 's1' - 2
}
// Main function - entry point of the program
int main()
{
cout << test("Hello") << endl; // Display the result of calling 'test' function with the string "Hello"
cout << test("Hi") << endl; // Display the result of calling 'test' function with the string "Hi"
cout << test("Python") << endl; // Display the result of calling 'test' function with the string "Python"
return 0; // Indicate successful completion of the program
}
Sample Output:
ell ytho
Visual Presentation:
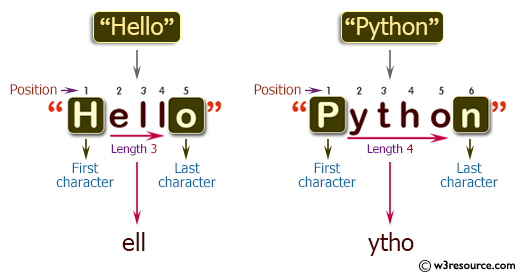
Flowchart:
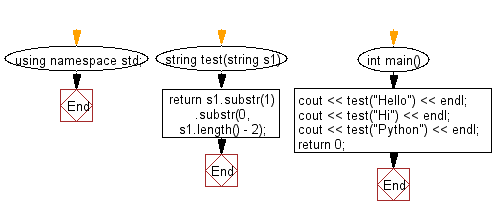
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string of the first half of a given string of even length.
Next: Write a C++ program to create a new string from two given string one is shorter and another is longer. The format of the new string will be long string + short string + long string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics