C++ Exercises: Create a new string using three copies of the last two character of a given string of length atleast two
C++ Basic Algorithm: Exercise-59 with Solution
Three Copies of Last Two Characters
Write a C++ program to create another string using three copies of the last two characters of a given string of length at least two.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' duplicates the last two characters of the input string
string test(string s1)
{
// Extracts the last two characters of the input string 's1'
string last2 = s1.substr(s1.length() - 2);
// Concatenates the extracted last two characters three times to form the final output
return last2 + last2 + last2;
}
// Main function to test the 'test' function
int main()
{
// Displays the output of the 'test' function for different string inputs
cout << test("Hello") << endl; // Output: "lololo" (duplicates "lo" three times)
cout << test("Hi") << endl; // Output: "HiHiHi" (duplicates "Hi" three times)
return 0;
}
Sample Output:
lololo HiHiHi
Visual Presentation:
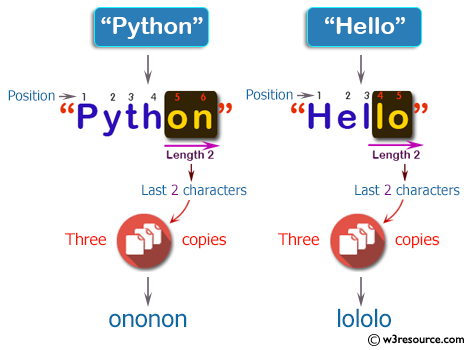
Flowchart:
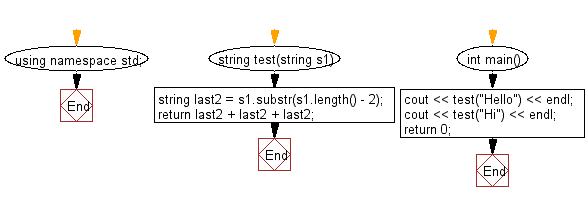
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to insert a given string into middle of the another given string of length 4.
Next: Write a C++ program to create a new string using first two characters of a given string. If the string length is less than 2 then return the original string.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-59.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics