C++ Exercises: Compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum
Sum Ignoring 13 and Right Values
Write a C++ program to compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes three integers (x, y, z) as parameters
int test(int x, int y, int z)
{
// Check if x is 13
if (x == 13)
return 0; // Return 0 if x is 13
// Check if y is 13
if (y == 13)
return x; // Return x if y is 13
// Check if z is 13
if (z == 13)
return x + y; // Return sum of x and y if z is 13
// If none of the above conditions are met, return the sum of x, y, and z
return x + y + z;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(4, 5, 7) << endl; // Output: 16 (x + y + z = 4 + 5 + 7 = 16)
cout << test(7, 4, 12) << endl; // Output: 23 (x + y + z = 7 + 4 + 12 = 23)
cout << test(10, 13, 12) << endl; // Output: 10 (y is 13, so returns x = 10)
cout << test(13, 12, 18) << endl; // Output: 0 (x is 13, so returns 0)
return 0;
}
Sample Output:
16 23 10 0
Visual Presentation:
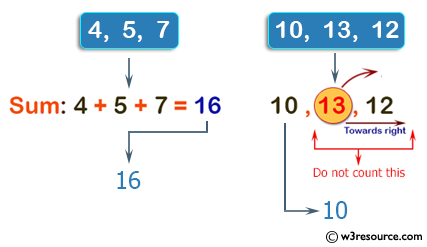
Flowchart:
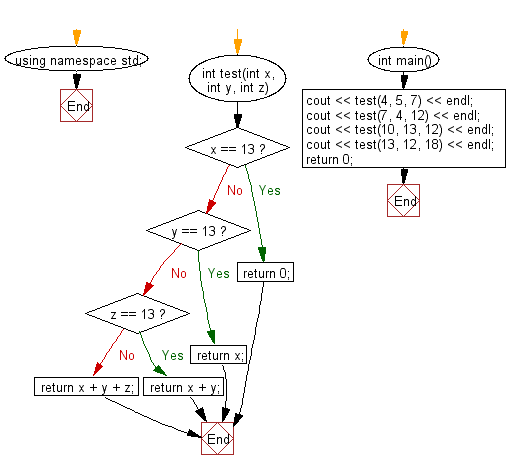
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of three integers, but if any value is 13, ignore it and any numbers to its right.
- Write a C++ program that reads three numbers and sums them while skipping the value 13 and any numbers following it in the sequence.
- Write a C++ program to add three integers with the condition that encountering 13 causes subsequent numbers not to be added to the sum.
- Write a C++ program that processes three inputs and, upon detecting a 13, omits it and any later numbers from the summation.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of three given integers. If the two values are same return the third value.
Next: Write a C++ program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
What is the difficulty level of this exercise?