C++ Exercises: Compute the sum of three given integers. If the two values are same return the third value
Sum or Third Integer if Two are Equal
Write a C++ program to compute the sum of three given integers. Return the third value if the two values are the same.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes three integers (x, y, z) as parameters
int test(int x, int y, int z)
{
// Check if x, y, and z are all equal
if (x == y && y == z)
return 0; // Return 0 if all three numbers are equal
// Check if x and y are equal
if (x == y)
return z; // Return z if x and y are equal
// Check if x and z are equal
if (x == z)
return y; // Return y if x and z are equal
// Check if y and z are equal
if (y == z)
return x; // Return x if y and z are equal
// If none of the above conditions are met, return the sum of x, y, and z
return x + y + z;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(4, 5, 7) << endl;
cout << test(7, 4, 12) << endl;
cout << test(10, 10, 12) << endl;
cout << test(12, 12, 18) << endl;
return 0;
}
Sample Output:
16 23 12 18
Visual Presentation:
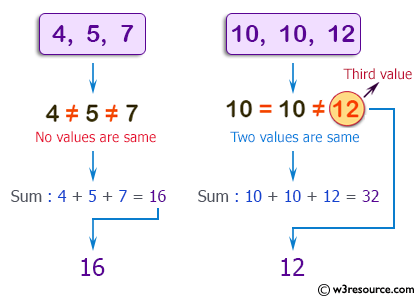
Flowchart:
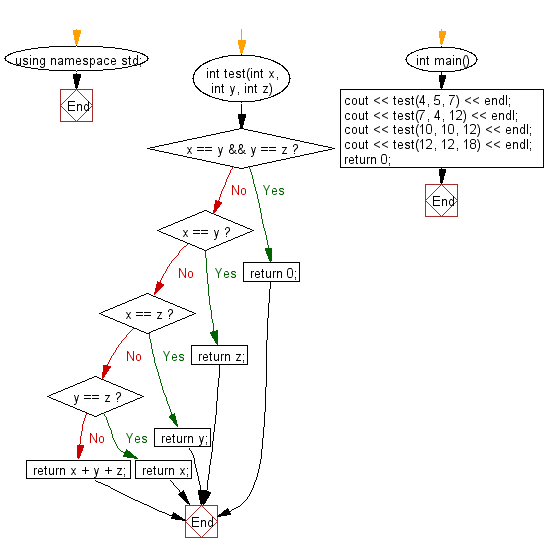
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x then return x without y.
Next: Write a C++ program to compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics