C++ Exercises: Check two given integers, each in the range 10..99
Shared Digit Between Two Integers (Range 10–99)
Write a C++ program to check two given integers. Each integer is in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes two integers (x, y) as parameters
bool test(int x, int y)
{
// Checks if the tens place of x equals the tens place of y
// OR if the tens place of x equals the ones place of y
// OR if the ones place of x equals the tens place of y
// OR if the ones place of x equals the ones place of y
return x / 10 == y / 10 || x / 10 == y % 10 || x % 10 == y / 10 || x % 10 == y % 10;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(11, 21) << endl;
cout << test(11, 20) << endl;
cout << test(10, 10) << endl;
return 0;
}
Sample Output:
1 0 1
Visual Presentation:
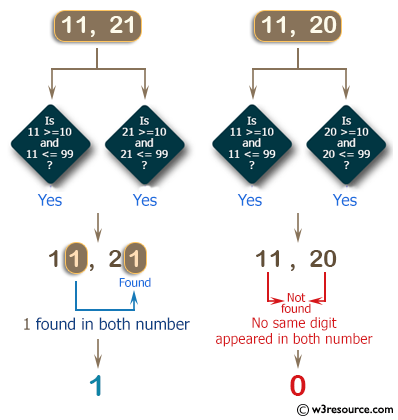
Flowchart:
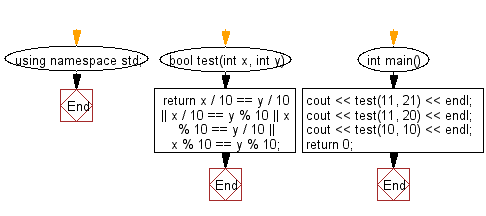
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check three given integers and return true if one of them is 20 or more less than one of the others.
Next: Write a C++ program to compute the sum of two given non-negative integers x and y as long as the sum has the same number of digits as x. If the sum has more digits than x then return x without y.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics