C++ Exercises: Check three given integers and return true if one of them is 20 or more less than one of the others
20 Less Than Another Integer
Write a C++ program to check three given integers and return true if one of them is 20 lower than one of the others.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes three integers (x, y, z) as parameters
bool test(int x, int y, int z)
{
// Returns true if the absolute difference between any two numbers among x, y, and z is greater than or equal to 20
return abs(x - y) >= 20 || abs(x - z) >= 20 || abs(y - z) >= 20;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(11, 21, 31) << endl; // Output: 1 (True, as differences between any two numbers are greater than or equal to 20)
cout << test(11, 22, 31) << endl; // Output: 1 (True, as differences between any two numbers are greater than or equal to 20)
cout << test(10, 20, 15) << endl; // Output: 0 (False, as no pair of numbers have differences greater than or equal to 20)
return 0;
}
Sample Output:
1 1 0
Visual Presentation:
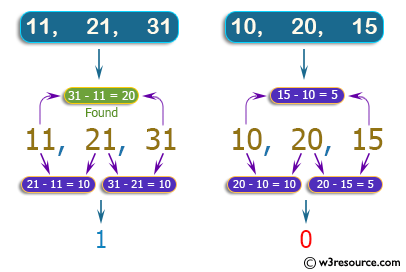
Flowchart:
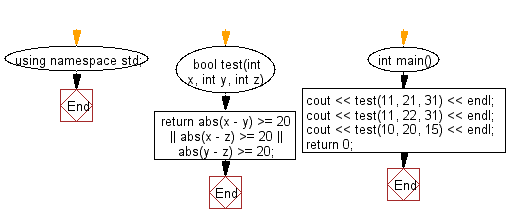
For more Practice: Solve these Related Problems:
- Write a C++ program to check three integers and return true if one of them is exactly 20 less than another.
- Write a C++ program that reads three numbers and verifies if any number is 20 lower than one of the others.
- Write a C++ program to compare three integers and output a boolean indicating whether the difference between any two equals 20.
- Write a C++ program that tests three input values and returns true if the difference between any pair is exactly 20.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if two or more non-negative given integers have the same rightmost digit.
Next: Write a C++ program to find the larger from two given integers. However if the two integers have the same remainder when divided by 7, then the return the smaller integer. If the two integers are the same, return 0.
What is the difficulty level of this exercise?