C++ Exercises: Check if two or more non-negative given integers have the same rightmost digit
Same Rightmost Digit for Non-Negative Integers
Write a C++ program to check if two or more integers that are not negative have the same rightmost digit.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function 'test' takes three integers (x, y, z) as parameters
bool test(int x, int y, int z)
{
// Returns true if any two numbers among x, y, and z have the same last digit
return x % 10 == y % 10 || x % 10 == z % 10 || y % 10 == z % 10;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(11, 21, 31) << endl; // Output: 1 (True, as two numbers end with the same digit '1')
cout << test(11, 22, 31) << endl; // Output: 1 (True, as two numbers end with the same digit '1')
cout << test(11, 22, 33) << endl; // Output: 0 (False, as no two numbers end with the same digit)
return 0;
}
Sample Output:
1 1 0
Visual Presentation:
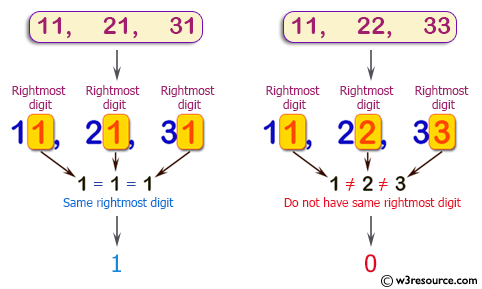
Flowchart:
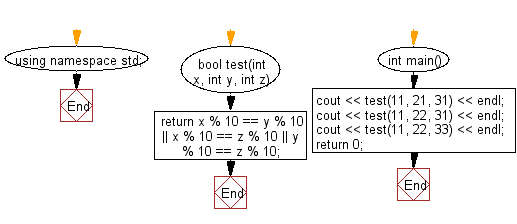
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if three given numbers are in strict increasing order, such as 4 7 15, or 45, 56, 67, but not 4 ,5, 8 or 6, 6, 8. However,if a fourth parameter is true, equality is allowed, such as 6, 6, 8 or 7, 7, 7.
Next: Write a C++ program to check three given integers and return true if one of them is 20 or more less than one of the others.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics