C++ Exercises: Check if y is greater than x, and z is greater than y from three given integers x,y,z
Check y > x and z > y
Write a C++ program to check if y is greater than x, and z is greater than y from three given integers x,y,z.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if x is less than y and y is less than z
bool test(int x, int y, int z)
{
return x < y && y < z;
}
int main()
{
// Testing the 'test' function with different sets of numbers
cout << test(1, 2, 3) << endl; // Output: 1 (True, as 1 < 2 < 3)
cout << test(4, 5, 6) << endl; // Output: 1 (True, as 4 < 5 < 6)
cout << test(-1, 1, 0) << endl; // Output: 0 (False, as -1 is not less than 0)
return 0;
}
Sample Output:
1 1 0
Visual Presentation:
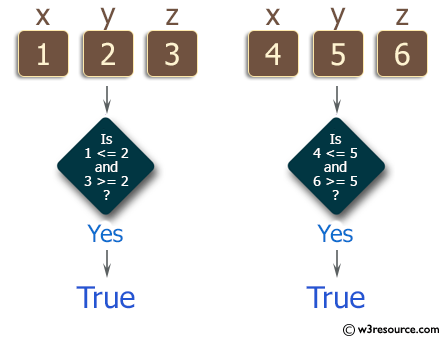
Flowchart:
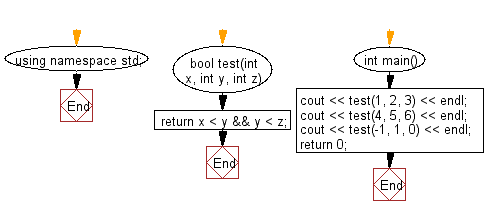
For more Practice: Solve these Related Problems:
- Write a C++ program to check whether three given integers are in strictly increasing order (i.e., x < y < z).
- Write a C++ program that reads three numbers and returns true if the second is greater than the first and the third is greater than the second.
- Write a C++ program to compare three integers and verify if they form an ascending sequence.
- Write a C++ program that accepts three values and outputs a boolean indicating whether they satisfy the condition x < y < z.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if it is possible to add two integers to get the third integer from three given integers.
Next: Write a C++ program to check if three given numbers are in strict increasing order, such as 4 7 15, or 45, 56, 67, but not 4 ,5, 8 or 6, 6, 8.However,if a fourth parameter is true, equality is allowed, such as 6, 6, 8 or 7, 7, 7.
What is the difficulty level of this exercise?