C++ Exercises: Compute the sum of the two given integers, if one of the given integer value is in the range 10..20 inclusive return 18
C++ Basic Algorithm: Exercise-42 with Solution
Return 18 if Integer in Range 10–20
Write a C++ program to compute the sum of the two given integers. Return 18 if one of the given integer values is in the range 10..20 inclusive.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to determine the result based on conditions involving two integers x and y
int test(int x, int y)
{
// If x or y is between 10 and 20 (inclusive), return 18, else return the sum of x and y
return (x >= 10 && x <= 20) || (y >= 10 && y <= 20) ? 18 : x + y;
}
int main()
{
// Testing the test function with different input values
cout << test(3, 7) << endl; // Output: 10 (sum of 3 and 7)
cout << test(10, 11) << endl; // Output: 18 (within the range 10-20)
cout << test(10, 20) << endl; // Output: 18 (within the range 10-20)
cout << test(21, 220) << endl; // Output: 241 (sum of 21 and 220, neither in the range 10-20)
return 0; // Return 0 to indicate successful completion
}
Sample Output:
10 18 18 241
Visual Presentation:
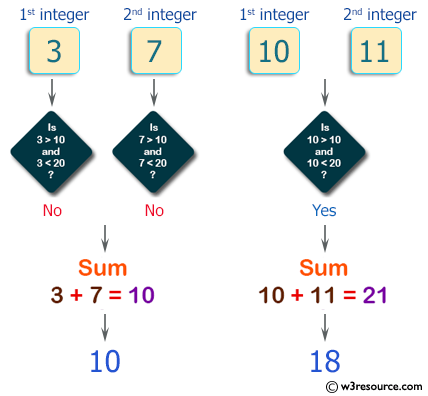
Flowchart:
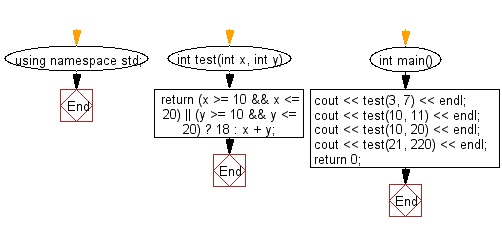
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given number is within 2 of a multiple of 10.
Next: Write a C++ program to check whether a given string starts with "F" or ends with "B". If the string starts with "F" return "Fizz" and return "Buzz" if it ends with "B" If the string starts with "F" and ends with "B" return "FizzBuzz". In other cases return the original string.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-42.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics