C++ Exercises: Check a given integer and return true if it is within 10 of 100 or 200
Within 10 of 100 or 200
Write a C++ program to check a given integer and return true if it is within 10 of 100 or 200.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the absolute difference between x and either 100 or 200 is less than or equal to 10
bool test(int x)
{
// If the absolute difference between x and 100 is less than or equal to 10 OR the absolute difference between x and 200 is less than or equal to 10
if (abs(x - 100) <= 10 || abs(x - 200) <= 10)
return true; // Return true if either condition is true
return false; // Return false if neither condition is true
}
// Main function
int main()
{
cout << test(103) << endl; // Output the result of test function with argument 103
cout << test(90) << endl; // Output the result of test function with argument 90
cout << test(89) << endl; // Output the result of test function with argument 89
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 0
Flowchart:
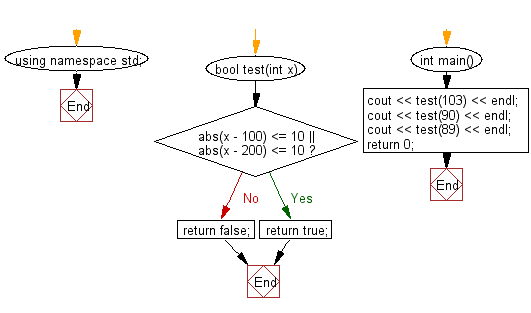
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Next: Write a C++ program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics