C++ Exercises: Accept two integers and return true if either one is 5 or their sum or difference is 5
C++ Basic Algorithm: Exercise-38 with Solution
Write a C++ program that accepts two integers and returns true if either one is 5 or their sum or difference is 5.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to evaluate conditions based on integer values x and y
bool test(int x, int y)
{
// Returns true if any of the conditions are satisfied:
// 1. x is equal to 5
// 2. y is equal to 5
// 3. The sum of x and y is equal to 5
// 4. The absolute difference between x and y is equal to 5
return x == 5 || y == 5 || x + y == 5 || abs(x - y) == 5;
}
int main()
{
// Test cases to check different scenarios of the test function
cout << test(5, 4) << endl; // Output: 1 (true)
cout << test(4, 3) << endl; // Output: 0 (false)
cout << test(1, 4) << endl; // Output: 1 (true)
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 0 1
Visual Presentation:
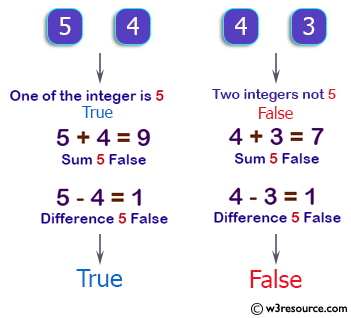
Flowchart:
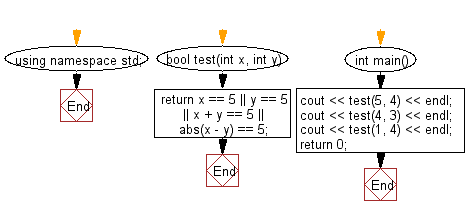
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Next: Write a C++ program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics