C++ Exercises: Check a given array of integers and return true if there are two values 15, 15 next to each other
Compare Strings for Same Length 2 Substrings
Write a C++ program to compare two given strings and return the number of positions where they contain the same length 2 substring.
Sample Input:
{ 5, 5, 1, 15, 15 }
{ 15, 2, 3, 4, 15 }
{ 3, 3, 15, 15, 5, 5}
{ 1, 5, 15, 7, 8, 15}
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the array contains consecutive occurrences of number 15
static bool test(int numbers[], int arr_length)
{
// Loop through the array elements (except the last one) to find consecutive occurrences of 15
for (int i = 0; i < arr_length - 1; i++)
{
// Check if the current element and the next element are both equal to 15
if (numbers[i + 1] == numbers[i] && numbers[i] == 15)
return true; // Return true if two consecutive elements are equal to 15
}
return false; // Return false if no consecutive occurrences of 15 are found
}
int main()
{
// Test cases with different arrays
int nums1[] = {5, 5, 1, 15, 15}; // Array 1
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculate the length of array 1
cout << test(nums1, arr_length) << endl; // Check if array 1 contains consecutive occurrences of 15
int nums2[] = {15, 2, 3, 4, 15}; // Array 2
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculate the length of array 2
cout << test(nums2, arr_length) << endl; // Check if array 2 contains consecutive occurrences of 15
int nums3[] = {3, 3, 15, 15, 5, 5}; // Array 3
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculate the length of array 3
cout << test(nums3, arr_length) << endl; // Check if array 3 contains consecutive occurrences of 15
int nums4[] = {1, 5, 15, 7, 8, 15}; // Array 4
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculate the length of array 4
cout << test(nums4, arr_length) << endl; // Check if array 4 contains consecutive occurrences of 15
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 0 1 0
Visual Presentation:
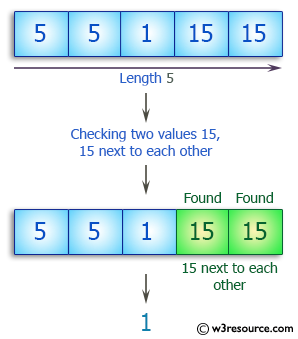
Flowchart:
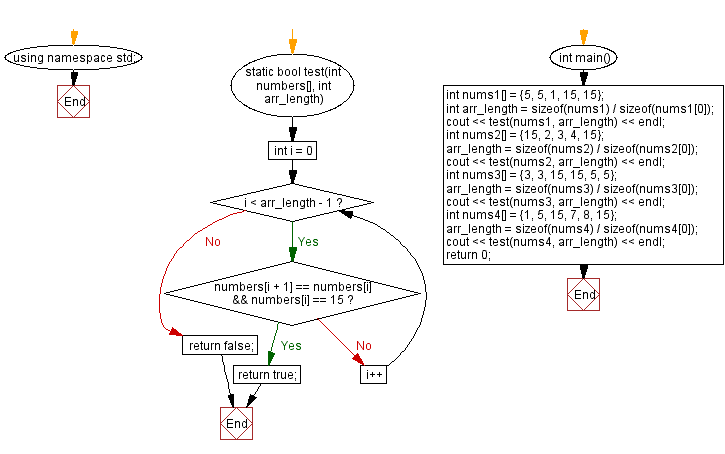
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Next: Write a C++ program to create a new string from a given string where a specified character have been removed except starting and ending position of the given string.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics