C++ Exercises: Count a substring of length 2 appears in a given string and also as the last 2 characters of the string
Count Substrings of Length 2
Write a C++ program to count the number of times a substring of length 2 appears in a given string as well as its last two characters. Do not count the end substring.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count occurrences of the last two characters as a substring in the input string
int test(string str)
{
// Extract the last two characters of the input string
string last_two_char = str.substr(str.length() - 2);
int ctr = 0; // Initialize a counter to zero
// Loop through the input string except for the last two characters
for (int i = 0; i < str.length() - 2; i++)
{
// Check if the substring of length 2 at index 'i' matches the last two characters
if (str.substr(i, 2) == (last_two_char))
ctr++; // Increment the counter for each match found
}
return ctr; // Return the count of occurrences of the last two characters as a substring
}
// Main function
int main()
{
// Output the results of the test function with different input strings
cout << test("abcdsab") << endl;
cout << test("abcdabab") << endl;
cout << test("abcabdabab") << endl;
cout << test("abcabd") << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
1 2 3 0
Visual Presentation:
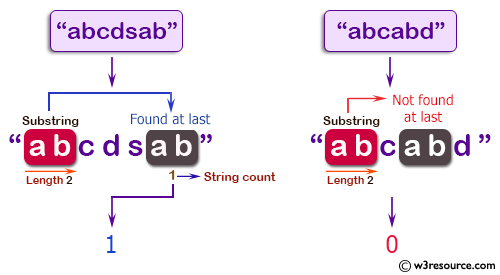
Flowchart:
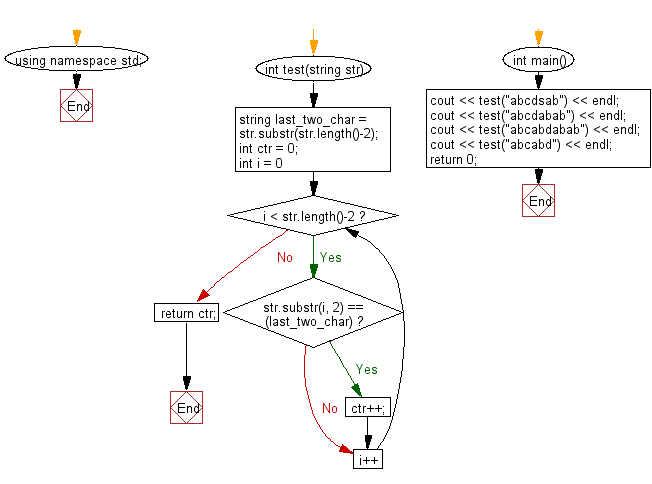
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a string like "aababcabcd" from a given string "abcd".
Next: Write a C++ program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics