C++ Exercises: Check two given integers, and return true if one of them is 30 or if their sum is 30
Check 30 or Sum 30
Write a C++ program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if either x is 30, y is 30, or the sum of x and y is 30
bool test(int x, int y)
{
return x == 30 || y == 30 || (x + y == 30); // Returns true if x is 30, y is 30, or the sum of x and y is 30; otherwise, returns false
}
// Main function
int main()
{
cout << test(30, 0) << endl; // Output the result of test function with arguments 30 and 0
cout << test(25, 5) << endl; // Output the result of test function with arguments 25 and 5
cout << test(20, 30) << endl; // Output the result of test function with arguments 20 and 30
cout << test(20, 25) << endl; // Output the result of test function with arguments 20 and 25
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 1 0
Visual Presentation:
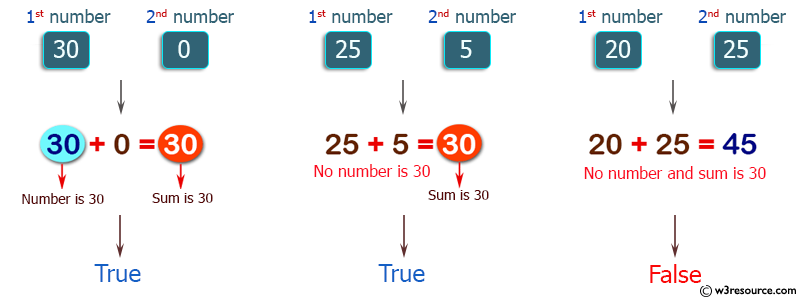
Flowchart:
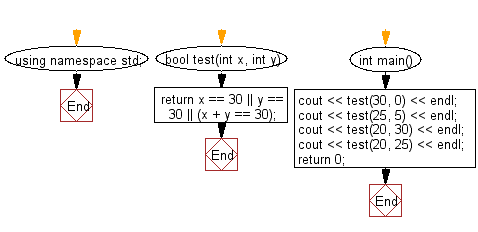
For more Practice: Solve these Related Problems:
- Write a C++ program that accepts two integers and returns true if either one is 30 or if their sum equals 30.
- Write a C++ program to check two numbers and output true if one number is exactly 30 or if adding them yields 30.
- Write a C++ program that reads two integer values and prints a boolean result indicating whether one of the numbers is 30 or their sum is 30.
- Write a C++ program to determine if either of the two input integers equals 30 or if their addition results in 30, then outputs a true/false value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
Next: Write a C++ program to check a given integer and return true if it is within 10 of 100 or 200.
What is the difficulty level of this exercise?