C++ Exercises: Create a new string made of every other character starting with the first from a given string
String with Every Other Character
Write a C++ program to create another string made of every other character starting with the first from a given string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to extract characters from the string at even positions
string test(string s)
{
string result = " "; // Initialize an empty string 'result'
// Loop through the characters of the input string 's'
for (int i = 0; i < s.length(); i++)
{
// Check if the current index 'i' is even
if (i % 2 == 0)
result += s[i]; // Append the character at even positions to 'result'
}
return result; // Return the resultant string 'result'
}
// Main function
int main()
{
// Output the results of the test function with different input strings
cout << test("Python") << endl; // Output: Pto (characters at even positions: P, t, o)
cout << test("PHP") << endl; // Output: PP (characters at even positions: P, P)
cout << test("JS") << endl; // Output: J (characters at even positions: J)
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Pto PP J
Visual Presentation:
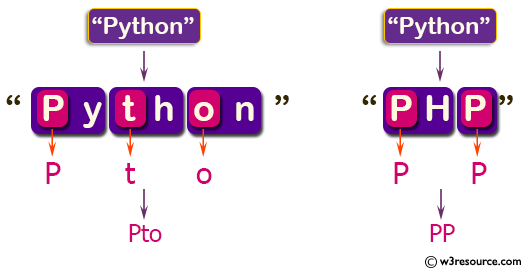
Flowchart:
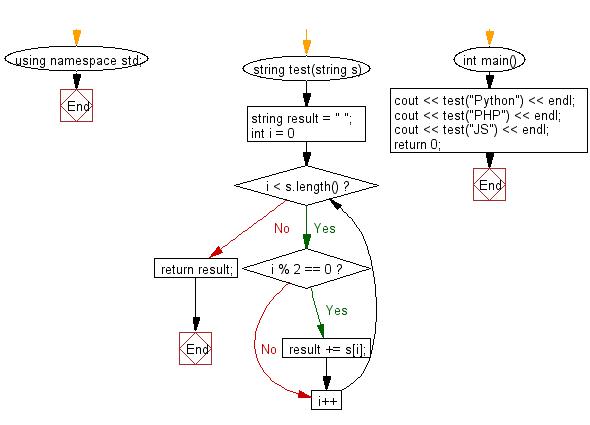
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if the first appearance of "a" in a given string is immediately followed by another "a".
Next: Write a C++ program to create a string like "aababcabcd" from a given string "abcd".
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics