C++ Exercises: Check if two given non-negative integers have the same last digit
Same Last Digit for Integers
Write a C++ program to check if two given non-negative integers have the same last digit.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the last digits of two numbers have the same absolute value
bool test(int x, int y)
{
// Return true if the absolute value of the last digit of both x and y are equal
return abs(x % 10) == abs(y % 10);
}
// Main function
int main()
{
// Output the result of the test function with different input numbers
cout << test(123, 456) << endl;
cout << test(12, 512) << endl;
cout << test(7, 87) << endl;
cout << test(12, 45) << endl;
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
0 1 1 0
Visual Presentation:
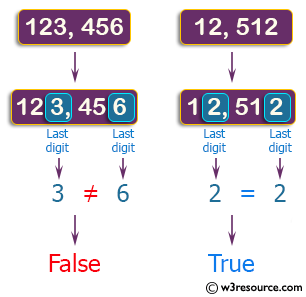
Flowchart:
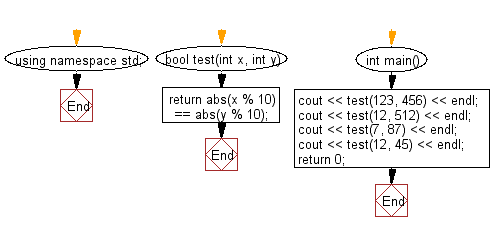
For more Practice: Solve these Related Problems:
- Write a C++ program to check if two non-negative integers have the same last digit by comparing their modulo 10 results.
- Write a C++ program that reads two integers and outputs true if their units digit is identical.
- Write a C++ program to determine if the last digit of two numbers matches, using modulus operations for the check.
- Write a C++ program that compares the final digit of two input numbers and prints true if they are the same.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given string contains between 2 and 4 'z' character.
Next: Write a C++ program to create a new string which is n (non-negative integer) copies of a given string.
What is the difficulty level of this exercise?