C++ Exercises: Check if a given string contains between 2 and 4 'z' character
C++ Basic Algorithm: Exercise-22 with Solution
Check 2–4 'z' Characters
Write a C++ program to check if a given string contains between 2 and 4 'z' characters.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count the occurrences of the character 'z' in the given string
bool test(string str)
{
int ctr = 0; // Counter variable to count occurrences of 'z'
// Loop through each character in the string
for (int i = 0; i < str.length(); i++)
{
// Check if the current character is 'z'
if (str[i] == 'z')
{
ctr++; // Increment the counter if 'z' is found
}
}
// Return true if the count of 'z' is more than 1 and less than 4, otherwise return false
return ctr > 1 && ctr < 4;
}
// Main function
int main()
{
// Output the result of the test function with different input strings
cout << test("frizz") << endl; // Output: 1 (true)
cout << test("zane") << endl; // Output: 0 (false)
cout << test("Zazz") << endl; // Output: 0 (false)
cout << test("false") << endl; // Output: 0 (false)
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 0 1 0
Visual Presentation:
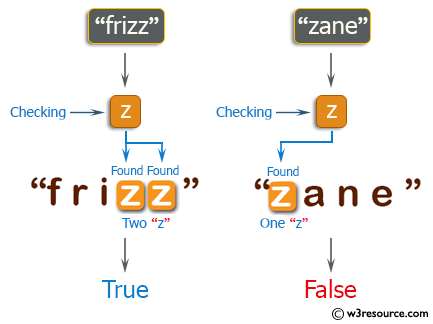
Flowchart:
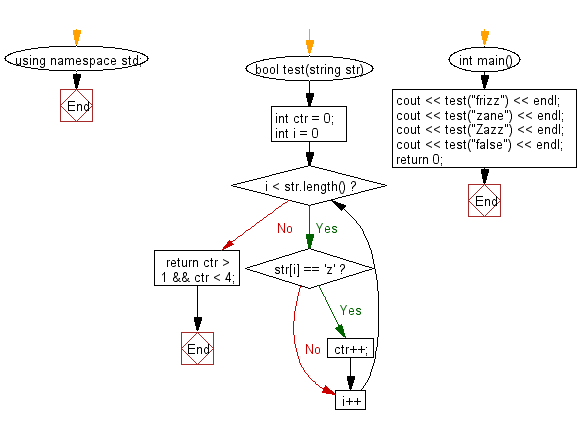
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
Next: Write a C++ program to check if two given non-negative integers have the same last digit.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics