C++ Exercises: Check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive
Check Two Integers in Ranges 40–50 or 50–60
Write a C++ program to check whether two given integers are in the range 40..50 inclusive, or they are both in the range 50..60 inclusive.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the given coordinates fall within specific ranges
bool test(int x, int y)
{
// Check if x and y fall within the specified ranges
return (x >= 40 && x <= 50 && y >= 40 && y <= 50) || (x >= 50 && x <= 60 && y >= 50 && y <= 60);
}
// Main function
int main()
{
cout << test(78, 95) << endl; // Output the result of test function with coordinates (78, 95)
cout << test(25, 35) << endl; // Output the result of test function with coordinates (25, 35)
cout << test(40, 50) << endl; // Output the result of test function with coordinates (40, 50)
cout << test(55, 60) << endl; // Output the result of test function with coordinates (55, 60)
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
0 0 1 1
Visual Presentation:
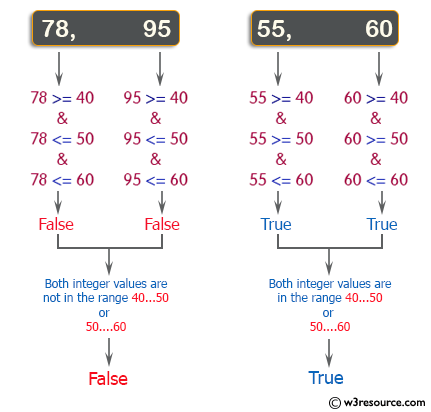
Flowchart:
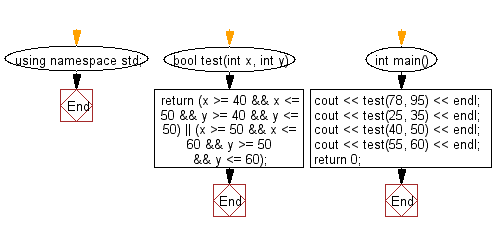
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Next: Write a C++ program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range..
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics