C++ Exercises: Check if a string 'yt' appears at index 1 in a given string
Remove 'yt' at Index 1
Write a C++ program to check if the string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to modify a string based on a substring condition
string test(string str)
{
// Check if the substring starting at index 1 and of length 2 equals "yt"
// If true, erase the substring from index 1 with length 2 from the original string
return str.substr(1, 2) == "yt" ? str.erase(1, 2) : str;
}
// Main function
int main()
{
cout << test("Python") << endl; // Output the result of test function with string "Python"
cout << test("ytade") << endl; // Output the result of test function with string "ytade"
cout << test("jsues") << endl; // Output the result of test function with string "jsues"
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
Phon ytade jsues
Visual Presentation:
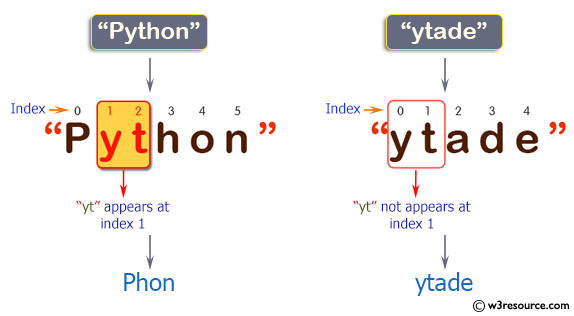
Flowchart:
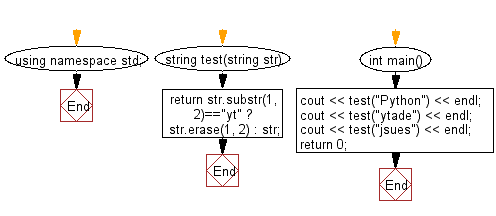
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
Next: Write a C++ program to check the largest number among three given integers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics