C++ Exercises: Check two given integers whether either of them is in the range 100..200 inclusive
Check if Integers are in Range 100–200
Write a C++ program to check two given integers whether either of them is in the range 100..200 inclusive.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if either of the two integers falls within the range 100 to 200 (inclusive)
bool test(int x, int y)
{
// Check if either x or y is within the range 100 to 200
return (x >= 100 && x <= 200) || (y >= 100 && y <= 200);
}
// Main function
int main()
{
cout << test(100, 199) << endl; // Output the result of test function with values 100 and 199
cout << test(250, 300) << endl; // Output the result of test function with values 250 and 300
cout << test(105, 190) << endl; // Output the result of test function with values 105 and 190
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 0 1
Visual Presentation:
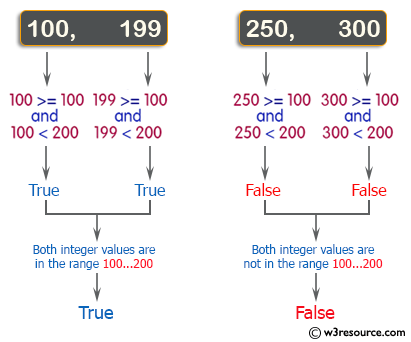
Flowchart:
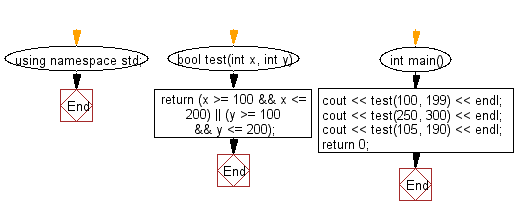
For more Practice: Solve these Related Problems:
- Write a C++ program to determine whether at least one of two integers lies in the inclusive range 100 to 200.
- Write a C++ program that checks two numbers and returns true if either one falls between 100 and 200, inclusive.
- Write a C++ program to test if one or both of the provided integers are within the range 100–200 and output a boolean value.
- Write a C++ program to validate two integer inputs and print true if any one of them is between 100 and 200, otherwise false.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if one given temperatures is less than 0 and the other is greater than 100.
Next: Write a C++ program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
What is the difficulty level of this exercise?