C++ Exercises: From a list of positive integers, create a list of their rightmost digits
Create List of Rightmost Digits of Numbers in Array
Write a C++ program to create a list of the rightmost digits from a given list of positive integers.
Test Data:
({ 12, 20, 351, 4449}) -> {2, 0, 1, 9}
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Includes all standard C++ libraries
#include <list> // Includes the list container
using namespace std; // Using standard namespace
// Function to create a new list with the rightmost digits of positive integers from the given list
list<int> test(list<int> nums) {
list<int> new_list; // Declare a new list to store modified elements
list<int>::iterator it; // Declare an iterator for traversing the input list 'nums'
// Iterate through 'nums' list, extract the rightmost digit of each positive integer, and store in 'new_list'
for (it = nums.begin(); it != nums.end(); ++it) {
new_list.push_back(*it % 10); // Extract the rightmost digit using the modulo operator
}
return new_list; // Return the new list with the rightmost digits
}
// Function to display the elements of a list
display_list(list<int> g) {
list<int>::iterator it; // Declare an iterator for traversing the input list 'g'
// Iterate through 'g' list and display each element
for (it = g.begin(); it != g.end(); ++it) {
cout << *it << ' '; // Print each element followed by a space
}
cout << '\n'; // Move to a new line after displaying all elements
}
int main() {
list<int> nums = {12, 20, 351 , 4449, 87654}; // Initialize a list 'nums' with positive integers
cout << "Original list of elements:\n";
display_list(nums); // Display the original list 'nums'
list<int> result_list; // Declare a list to store the result of test() function
result_list = test(nums); // Call the test() function to extract rightmost digits
cout << "\nNew list of the rightmost digits from \nthe said list of positive integers:\n";
display_list(result_list); // Display the new list with rightmost digits
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
Original list of elements: 12 20 351 4449 87654 New list of the rightmost digits from the said list of positive integers: 2 0 1 9 4
Flowchart:
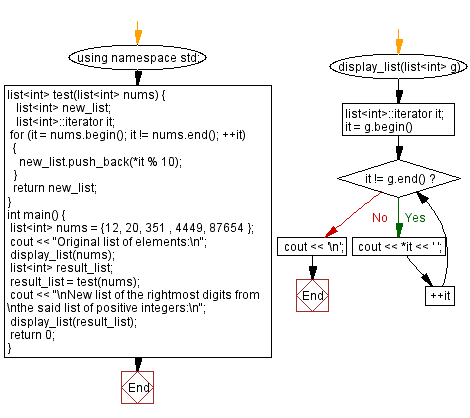
For more Practice: Solve these Related Problems:
- Write a C++ program to generate a new array that contains the last digit (units place) of each integer from the input array.
- Write a C++ program that reads an array of positive integers and outputs a new list with each element replaced by its rightmost digit.
- Write a C++ program to process an integer array and extract the units digit from each number, then print the resulting array.
- Write a C++ program that takes an array of integers and creates a new array consisting solely of the last digit of each original number.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Create a list of integers, add each value to 3 and multiply the result by 4.
What is the difficulty level of this exercise?