C++ Exercises: Create a list of integers, add each value to 3 and multiply the result by 4
C++ Basic Algorithm: Exercise-128 with Solution
Write a C++ program to create a list from a given list of integers. In this program, each integer value is added to 3 and the result value is multiplied by 4.
Test Data:
({ 1, 2, 3 , 4 }) -> {16 20 24 28}
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard C++ libraries
#include <list> // Include the list container
using namespace std; // Using standard namespace
// Function to create a new list where each integer value is added to 3 and the result value is multiplied by 4
list<int> test(list<int> nums) {
list<int> new_list; // Declare a new list to store modified elements
list<int>::iterator it; // Declare an iterator for traversing the input list 'nums'
// Iterate through 'nums' list, perform arithmetic operations, and store the modified values in 'new_list'
for (it = nums.begin(); it != nums.end(); ++it) {
new_list.push_back((*it + 3) * 4); // Perform addition and multiplication operations on each integer
}
return new_list; // Return the new list with modified elements
}
// Function to display the elements of a list
display_list(list<int> g) {
list<int>::iterator it; // Declare an iterator for traversing the input list 'g'
// Iterate through 'g' list and display each element
for (it = g.begin(); it != g.end(); ++it) {
cout << *it << ' '; // Print each element followed by a space
}
cout << '\n'; // Move to a new line after displaying all elements
}
int main() {
list<int> nums = {1, 2, 3, 4, 5, 6, 7, 8, 9}; // Initialize a list 'nums' with integers
cout << "Original list of elements:\n";
display_list(nums); // Display the original list 'nums'
list<int> result_list; // Declare a list to store the result of test() function
result_list = test(nums); // Call the test() function to perform arithmetic operations on each integer
cout << "\nNew list from the said where each integer value is \nadded to 3 and the result value is multiplied by 4:\n";
display_list(result_list); // Display the new list with modified elements
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
Original list of elements: 1 2 3 4 5 6 7 8 9 New list from the said where each integer value is added to 3 and the result value is multiplied by 4: 16 20 24 28 32 36 40 44 48
Flowchart:
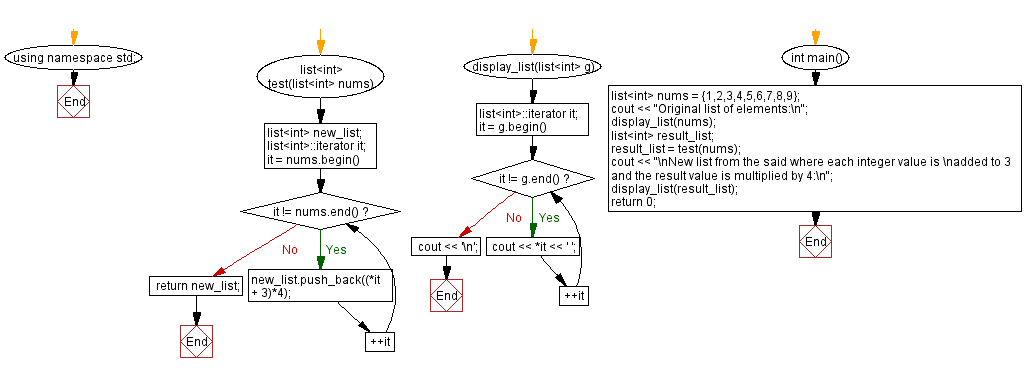
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Concatenate three copies of each string in a given list of strings.
Next: From a list of positive integers, create a list of their rightmost digits.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics