C++ Exercises: Create an array of odd numbers and specific lengths from an array
Create Array of Odd Numbers with Specific Length
Write a C++ program that creates a new array of odd numbers with specific lengths from a given array of positive integers.
Test Data:
({1,2,3,5,7,9,10},3) -> {1,3,5}
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include <string.h> // Including string manipulation header file
using namespace std; // Using standard namespace
int *odds; // Declaration of an integer pointer variable
// Function to filter odd numbers from an array
int *test(int *nums, int n) {
odds = new int[n]; // Allocating memory for 'odds' array
int j = 0; // Initializing variable 'j' to 0
for (int i = 0; j < n; i++) {
if (nums[i] % 2 != 0) {
odds[j] = nums[i]; // Storing odd numbers in the 'odds' array
j++;
}
}
return odds; // Returning the array containing odd numbers
}
// Main function
int main() {
int nums[] = {1, 2, 3, 4, 5, 6, 7, 8, 9}; // Initializing an array of integers
int *result_array; // Declaration of an integer pointer variable
int arr_length = sizeof(nums) / sizeof(nums[0]); // Calculating the length of the array
// Displaying the original array elements
cout << "Original array elements: " << endl;
for (int i = 0; i < arr_length; i++) {
std::cout << nums[i] << ' ';
}
int n = 3; // Setting the length of the new array to store odd numbers
odds = test(nums, n); // Filtering odd numbers from 'nums' array and storing in 'odds' array
cout << "\n\nNew array of odd numbers " << "(length = " << n << ")" << " from the said array: " << endl;
// Displaying the new array containing odd numbers of specified length
for (int i = 0; i < n; i++) {
std::cout << odds[i] << ' ';
}
n = 5; // Updating the length of the new array
odds = test(nums, n); // Filtering odd numbers from 'nums' array and storing in 'odds' array
cout << "\n\nNew array of odd numbers " << "(length = " << n << ")" << " from the said array: " << endl;
// Displaying the new array containing odd numbers of updated length
for (int i = 0; i < n; i++) {
std::cout << odds[i] << ' ';
}
delete[] odds; // Deallocating memory allocated for 'odds' array
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array elements: 1 2 3 4 5 6 7 8 9 New array of odd numbers (length = 3) from the said array: 1 3 5 New array of odd numbers (length = 5) from the said array: 1 3 5 7 9
Flowchart:
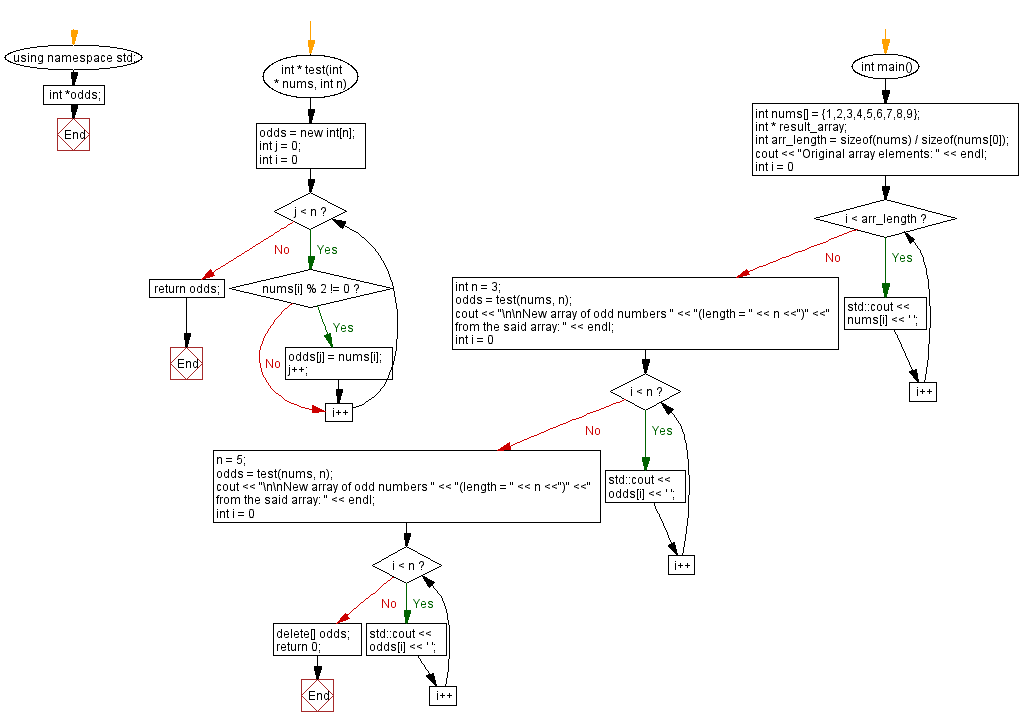
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Test a positive integer and return true if it contains the number 2.
Next: Each element of a given list of integers is multiplied by five.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics