C++ Exercises: New array from an existing array
Create Array of First n Strings from Array
Write a C++ program to create an array using the first n strings from a given array of strings. (n>=1 and <=length of the array).
Test Data:
({"a", "b", "bb", "c", "ccc" }, 2) -> {"a", "b"}
({"a", "b", "bb", "c", "ccc" }, 3) -> {"a", "b", "bb"}
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
#include<string.h> // Including string manipulation header file
using namespace std; // Using standard namespace
string *new_array; // Declaring a pointer for the new string array
// Function to create a new string array and copy elements from the original array
string *test(string *text, int n) {
new_array = new string[n]; // Creating a new string array of size 'n'
// Loop to copy elements from the original array 'text' to the new array 'new_array'
for(int i = 0; i < n; i++) {
new_array[i] = text[i]; // Copying each element from 'text' to 'new_array'
}
return new_array; // Returning the pointer to the new string array
}
// Main function
int main() {
string text[] = {"a", "b", "bb", "c", "ccc"}; // Original array of strings
string* result_array; // Pointer to store the returned new string array
int arr_length = sizeof(text) / sizeof(text[0]); // Calculating the length of the original array
cout << "Original array elements: " << endl;
// Displaying the original array elements
for (int i = 0; i < arr_length; i++) {
std::cout << text[i] << ' ';
}
int n = 2; // Size of the new array
result_array = test(text, n); // Calling 'test' function to create a new array of size 'n'
// Displaying the new array with size 'n'
cout << "\n\nNew array with size " << n << endl;
for(int i = 0; i < n; i++) {
std::cout << result_array[i] << ' ';
}
n = 4; // Changing the size of the new array
result_array = test(text, n); // Calling 'test' function again with a different size
// Displaying the new array with size 'n'
cout << "\n\nNew array with size " << n << endl;
for(int i = 0; i < n; i++) {
std::cout << result_array[i] << ' ';
}
delete [] new_array; // Deleting the allocated memory for the new array
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array elements: a b bb c ccc New array with size 2 a b New array with size 4 a b bb c
Flowchart:
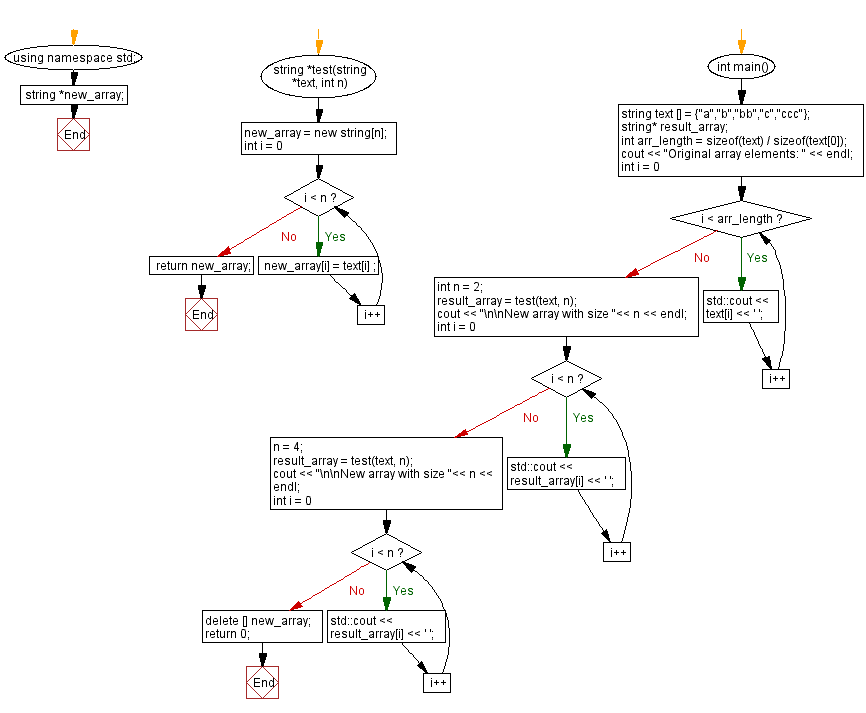
For more Practice: Solve these Related Problems:
- Write a C++ program to create a new array containing the first n strings from an existing string array.
- Write a C++ program that reads an array of strings and an integer n, then outputs an array of the first n strings.
- Write a C++ program to extract the initial n elements from a string array and display them as a new array.
- Write a C++ program that processes a string array and returns a subarray composed of its first n entries, where n is provided by the user.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Count the number of strings with a given length in an array.
Next: Array from an existing array using string length.
What is the difficulty level of this exercise?