C++ Exercises: Find the largest average value between the first and second halves of a given array of integers
Find Larger Average Between First and Second Halves of Array
Write a C++ program to find the largest average value between the first and second halves of a given array of integers. Ensure that the minimum length is at least 2. Assume that the second half begins at index (array length)/2.
Test Data:
({ 1, 2, 3, 4, 6, 8 }) -> 6
({ 15, 2, 3, 4, 15, 11 }) -> 10
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Forward declaration of function avgg
int avgg(int[], int, int, size_t);
// Function to find the larger average value between the first and second halves of an array
int test(int numbers[], int arr_length) {
// Finding the average of the first half
int firstHalf = avgg(numbers, 0, arr_length / 2, arr_length);
// Finding the average of the second half
int secondHalf = avgg(numbers, arr_length / 2, arr_length, arr_length);
// Returning the larger of the two averages
return firstHalf > secondHalf ? firstHalf : secondHalf;
}
// Function to calculate the average of elements within a specified range
int avgg(int num[], int start, int end, size_t arr_length) {
int sum = 0;
// Calculating the sum of elements within the range
for (int i = start; i < end; i++)
sum += num[i];
// Calculating and returning the average
return sum / (arr_length / 2);
}
// Main function
int main() {
// First test array
int nums1[] = {1, 2, 3, 4, 6, 8 };
size_t arr_length = sizeof(nums1) / sizeof(int);
// Displaying original array elements
cout << "Original array elements: ";
for (size_t i = 0; i < arr_length; i++) {
std::cout << nums1[i] << ' ';
}
// Finding and displaying the larger average value between the first and second halves
cout << "\nLarger average value between the first and the second half of the said array: ";
cout << test(nums1, arr_length) << endl;
// Second test array
int nums2[] = { 15, 2, 3, 4, 15, 11 };
arr_length = sizeof(nums2) / sizeof(int);
// Displaying original array elements
cout << "\nOriginal array elements: ";
for (size_t i = 0; i < arr_length; i++) {
std::cout << nums2[i] << ' ';
}
// Finding and displaying the larger average value between the first and second halves
cout << "\nLarger average value between the first and the second half of the said array: ";
cout << test(nums2, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Original array elements: 1 2 3 4 6 8 Larger average value between the first and the second half of the said array: 6 Original array elements: 15 2 3 4 15 11 Larger average value between the first and the second half of the said array: 10
Flowchart:
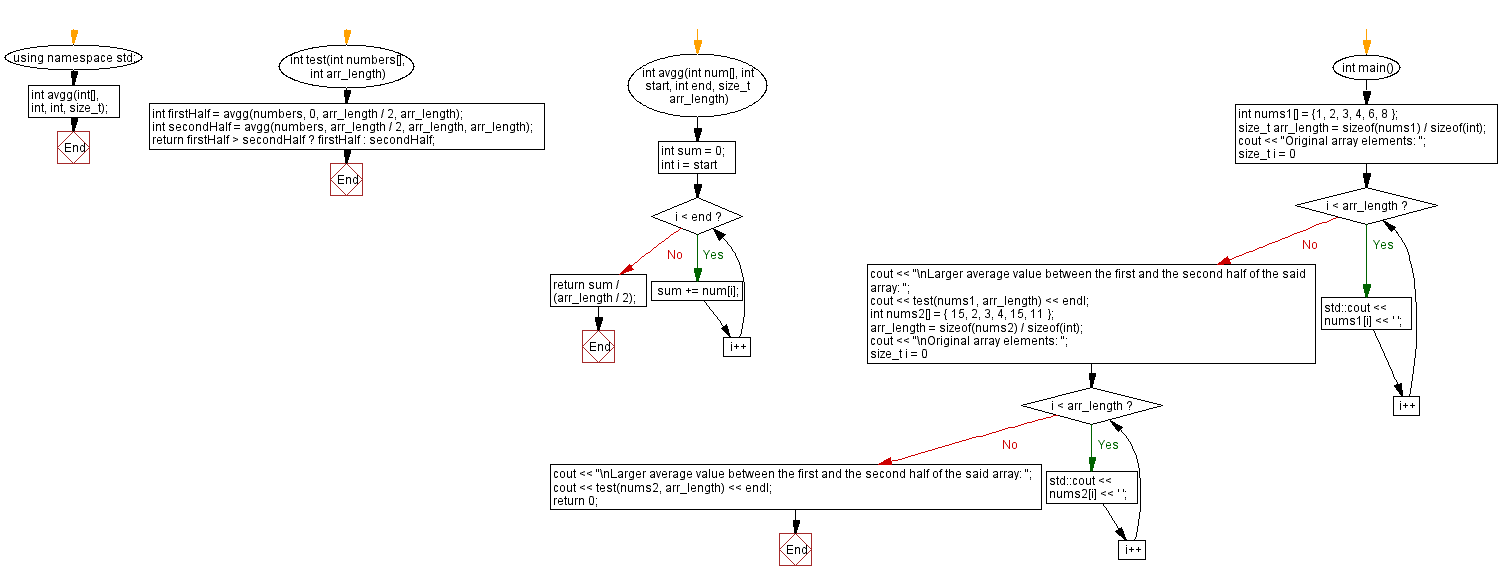
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Check if there are two values 15, 15 adjacent to each other in a given array of integers.
Next: Count the number of strings with a given length in an array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics