C++ Exercises: Check if the value of each element is equal or greater than the value of previous element of a given array of integers
Check if Array is Non-Decreasing
Write a C++ program to check if the value of each element is equal or greater than the value of the previous element of a given array of integers.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if the elements in the array are in ascending order
static bool test(int numbers[], int arr_length)
{
for (int i = 0; i < arr_length - 1; i++)
{
if (numbers[i + 1] < numbers[i]) return false; // If the next element is smaller than the current element, return false
}
return true; // Return true if all elements are in ascending order
}
// Main function
int main()
{
// Different test cases with arrays of integers
int nums1[] = {5, 5, 1, 5, 5}; // Not in ascending order
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 2, 3, 4 }; // In ascending order
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 5, 5, 5, 5}; // In ascending order
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
int nums4[] = {1, 5, 5, 7, 8, 10}; // In ascending order
arr_length = sizeof(nums4) / sizeof(nums4[0]);
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
0 1 1 1
Flowchart:
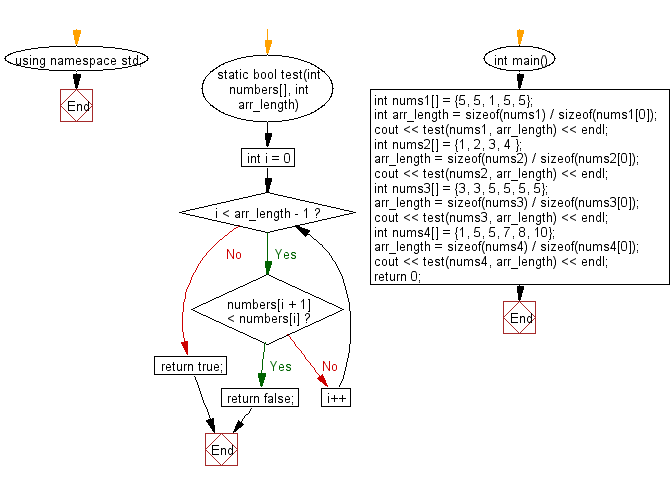
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Next: Check if there are two values 15, 15 adjacent to each other in a given array of integers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics