C++ Exercises: Check a given array of integers and return true if the array contains three increasing adjacent numbers
Check for Three Increasing Adjacent Numbers in Array
Write a C++ program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if there are three consecutive elements in the array that are in increasing order
static bool test(int numbers[], int arr_length)
{
for (int i = 0; i <= arr_length - 3; i++)
{
// Checking if the current element is one less than the next and also one less than the one after that
if (numbers[i] == numbers[i + 1] - 1 && numbers[i] == numbers[i + 2] - 2)
{
return true; // If found, return true
}
}
return false; // Return false if no such sequence is found
}
// Main function
int main()
{
// Different test cases with arrays of integers
int nums1[] = {1, 2, 3, 5, 3, 7}; // Contains a sequence of 3 consecutive numbers (1, 2, 3)
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {3, 7, 5, 5, 3, 7 }; // Does not contain a sequence of 3 consecutive numbers
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 7, 5, 5, 6, 7, 5}; // Contains a sequence of 3 consecutive numbers (5, 6, 7)
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1
Visual Presentation:
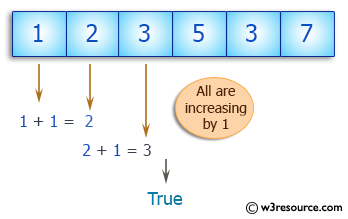
Flowchart:
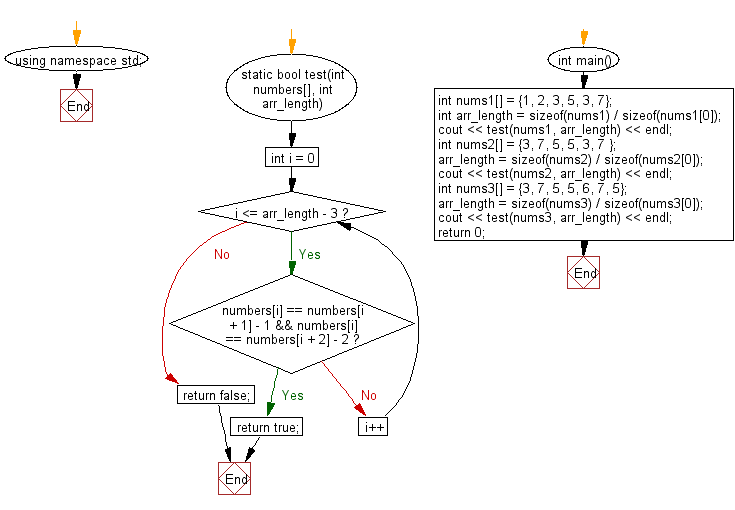
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array.
Next: Write a C++ program to check if the value of each element is equal or greater than the value of previous element of a given array of integers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics