C++ Exercises: Check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array
Check if Specified Elements Appear at Both Start and End of Array
Write a C++ program to check a given array of integers. The program will return true if the specified number of the same elements appears at the start and end of the given array.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if the last 'n' elements in the array match the first 'n' elements
static bool test(int numbers[], int arr_length, int n)
{
// Loop through the first 'n' elements and compare with the last 'n' elements
for (int i = 0; i < n; i++)
{
// Check for inequality between elements from the beginning and end of the array
if (numbers[i] != numbers[arr_length - n + i])
{
return false; // Return false if inequality is found
}
}
return true; // Return true if all 'n' elements match between the two segments
}
// Main function
int main()
{
// Different test cases with arrays of integers and 'n' value
int nums1[] = {3, 7, 5, 5, 3, 7 }; // Last 2 elements match the first 2 elements
int n = 2;
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length, n) << endl;
int nums2[] = {3, 7, 5, 5, 3, 7}; // Last 3 elements do not match the first 3 elements
n = 3;
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length, n) << endl;
int nums3[] = {3, 7, 5, 5, 3, 7, 5}; // Last 3 elements match the first 3 elements
n = 3;
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length, n) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1
Visual Presentation:
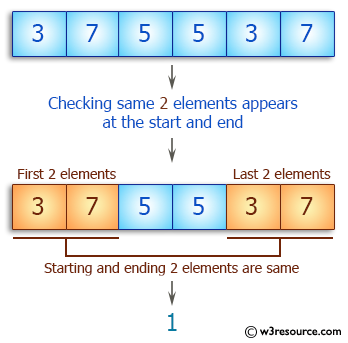
Flowchart:
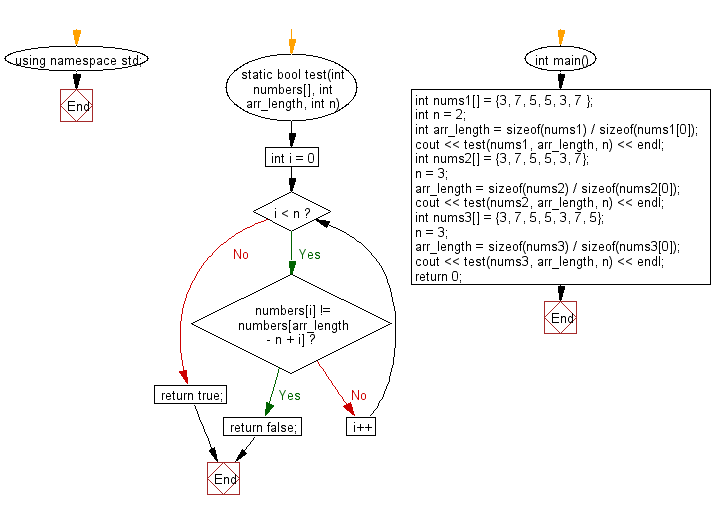
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a specified number of identical elements appear consecutively at both the beginning and the end of an array.
- Write a C++ program that reads an array and an integer n, then returns true if the first n elements match the last n elements.
- Write a C++ program to determine if an array’s first n elements are identical to its last n elements, and print the corresponding boolean value.
- Write a C++ program that compares the starting and ending segments (of length n) of an integer array and returns true if they are the same.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if every 5 that appears in the given array is next to another 5.
Next: Write a C++ program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
What is the difficulty level of this exercise?