C++ Exercises: Check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other
Check if Five '5's Exist Without Adjacent Ones
Write a C++ program to check a given array of integers. The program will return true if the value 5 appears 5 times and there are no 5 next to each other.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks if the number 5 appears exactly 5 times consecutively in the array
static bool test(int numbers[], int arr_length)
{
bool flag = false; // Flag to indicate if a sequence of 5s is encountered
int five = 0; // Counter to track the number of occurrences of 5
// Loop through the array to find sequences of consecutive 5s
for (int i = 0; i < arr_length; i++)
{
// Checking for the occurrence of 5 and handling the flag for consecutive 5s
if (numbers[i] == 5 && !flag)
{
five++; // Increment the count of 5s
flag = true; // Set flag to true indicating the start of a sequence of 5s
}
else
{
flag = false; // Reset flag if the sequence is interrupted
}
}
// Return true if the number 5 appears exactly 5 times consecutively, otherwise false
return five == 5;
}
int main()
{
// Different test cases with arrays of integers
int nums1[] = {3, 5, 1, 5, 3, 5, 7, 5, 1, 5}; // 5 appears exactly 5 times consecutively
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {3, 5, 5, 5, 5, 5, 5}; // 5 appears more than 5 times consecutively
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 5, 2, 5, 4, 5, 7, 5, 8, 5}; // 5 appears more than 5 times consecutively
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
int nums4[] = {2, 4, 5, 5, 5, 5}; // 5 appears exactly 5 times consecutively
arr_length = sizeof(nums4) / sizeof(nums4[0]);
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 0
Visual Presentation:
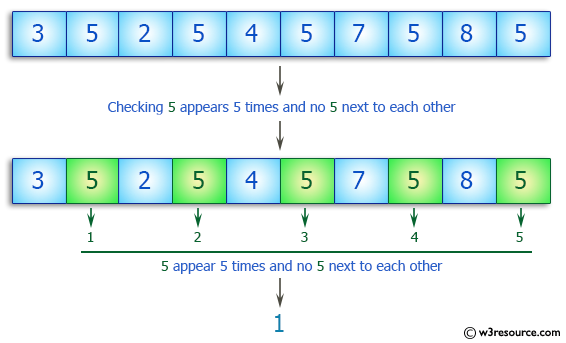
Flowchart:
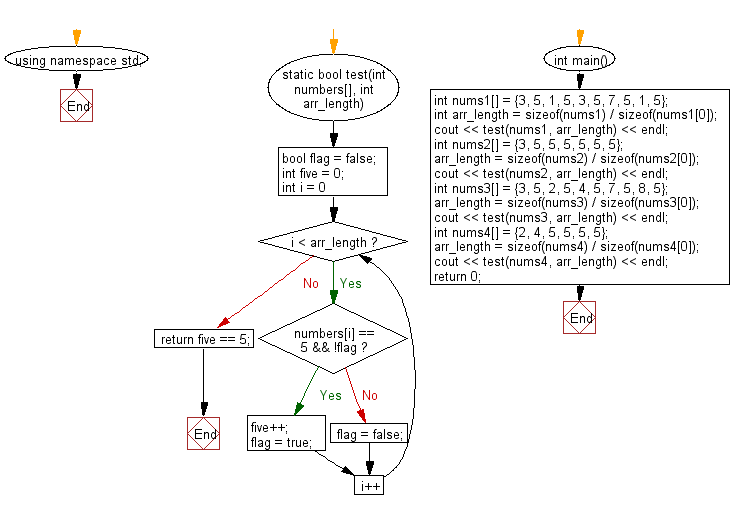
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other.
Next: Write a C++ program to check a given array of integers and return true if every 5 that appears in the given array is next to another 5.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics