C++ Exercises: Check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array
C++ Basic Algorithm: Exercise-110 with Solution
Check if '3' is Followed by '5' in Array
Write a C++ program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks for the occurrence of '5' following '3' in the array
static bool test(int nums[], int arr_length)
{
bool three = false; // Initializing a boolean variable 'three' to keep track of '3' occurrence
// Loop through the array elements to find a '5' following '3'
for (int i = 0; i < arr_length; i++)
{
// If 'three' is true (meaning '3' was previously encountered) and current element is '5', return true
if (three && nums[i] == 5) return true;
// If current element is '3', set 'three' to true to track its occurrence
if (nums[i] == 3) three = true;
}
return false; // Return false if '5' following '3' is not found in the array
}
int main()
{
int nums1[] = {3, 5, 1, 3, 7}; // Initializing array nums1 with '5' following '3'
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result (true or false)
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 2, 3, 4}; // Initializing array nums2 with no '5' following '3'
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result (true or false)
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 5, 5, 5, 5}; // Initializing array nums3 with '5' following '3'
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculating length of array nums3
// Calling test function with nums3 and displaying the result (true or false)
cout << test(nums3, arr_length) << endl;
int nums4[] = {2, 5, 5, 7, 8, 10}; // Initializing array nums4 with no '5' following '3'
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculating length of array nums4
// Calling test function with nums4 and displaying the result (true or false)
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 0
Visual Presentation:
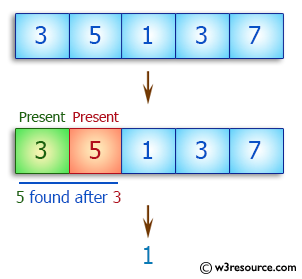
Flowchart:
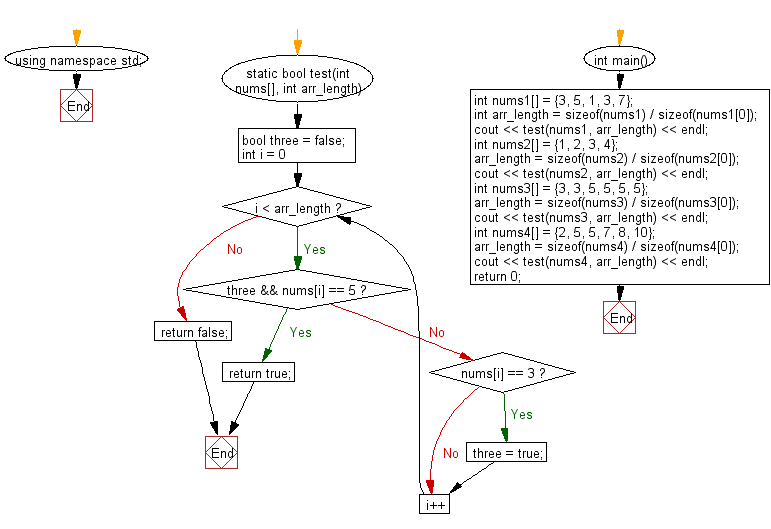
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if an array of integers contains a 3 next to a 3 or a 5 next to a 5 or both.
Next: Write a C++ program to check a given array of integers and return true if the given array contains either 2 even or 2 odd values all next to each other.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-110.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics