C++ Exercises: Check a given array of integers and return true if the given array contains two 5's next to each other, or two 5 separated by one element
Check if Array Contains Two '5's Next or Separated by One Element
Write a C++ program to check a given array of integers. Then, return true if the given array contains two 5's next to each other, or two 5's separated by one element.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks for the presence of '5' twice consecutively in the array
static bool test(int nums[], int arr_length)
{
int len = arr_length; // Storing the length of the array in 'len'
// Loop through the array elements to check for specific '5' patterns
for (int i = 0; i < len - 1; i++)
{
// Checking if '5' appears twice consecutively at i and i+1 indices
if (nums[i] == 5 && nums[i + 1] == 5) return true;
// Checking if '5' appears twice consecutively but with one element in between
if (i + 2 < len && nums[i] == 5 && nums[i + 2] == 5) return true;
}
return false; // Returning false if the specified '5' patterns are not found
}
int main()
{
int nums1[] = {5, 5, 1, 5, 5}; // Initializing array nums1 with specific '5' patterns
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result (true or false)
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 2, 3, 4}; // Initializing array nums2 with no specific '5' patterns
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result (true or false)
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 5, 5, 5, 5}; // Initializing array nums3 with specific '5' patterns
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculating length of array nums3
// Calling test function with nums3 and displaying the result (true or false)
cout << test(nums3, arr_length) << endl;
int nums4[] = {1, 5, 5, 7, 8, 10}; // Initializing array nums4 with no specific '5' patterns
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculating length of array nums4
// Calling test function with nums4 and displaying the result (true or false)
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 1
Visual Presentation:
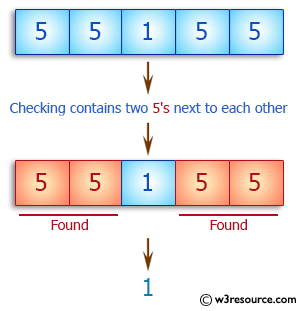
Flowchart:
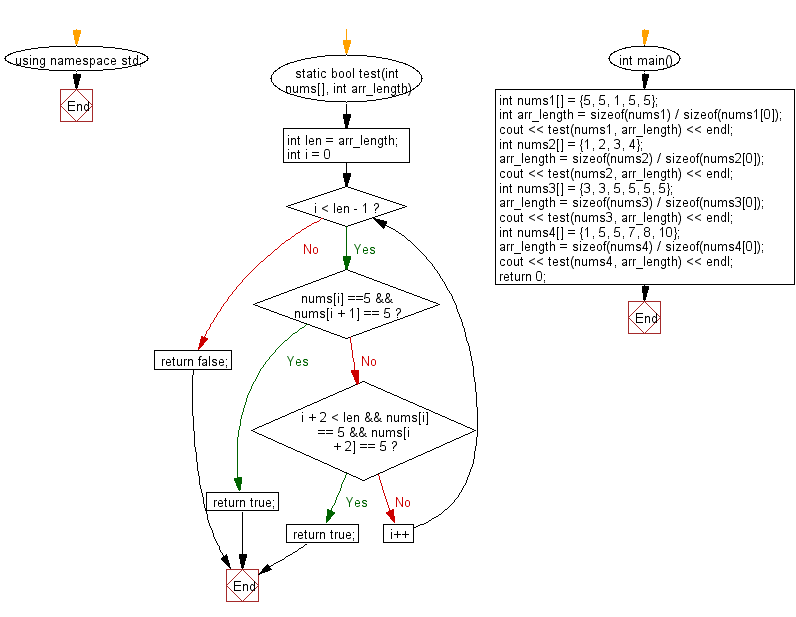
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if an array of integers contains a 3 next to a 3 or a 5 next to a 5 or both.
Next: Write a C++ program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics