C++ Exercises: Compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6
Sum Array Excluding Numbers Starting with '5' and Followed by '6'
Write a C++ program to compute the sum of the numbers in a given array except the ones starting with 5 followed by at least one 6. Return 0 if the given array has no integers.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that calculates the sum of numbers in the array
// excluding those numbers starting with 5 followed by at least one 6
static int test(int nums[], int arr_length)
{
int sum = 0; // Initializing variable to store the sum
int inSection = 0; // Variable to determine if within the specified section
int flag = 0; // Flag to identify the start of the specified section
// Loop through the array to calculate the sum following the specified conditions
for (int i = 0; i < arr_length; i++)
{
inSection = 0; // Resetting inSection flag for each iteration
// Checking if the current number is 5 to potentially begin the specified section
if (nums[i] == 5)
{
inSection = 0; // Resetting inSection flag
flag = 1; // Setting flag to mark the start of the specified section
}
// Checking for conditions to identify the section and adjusting the sum accordingly
if (inSection == 0 && nums[i] == 6)
{
if (flag == 1) // If the start of the section was flagged by 5
{
sum = sum - 5; // Subtracting the previous 5 before 6
inSection = 1; // Marking within the specified section
}
flag = 0; // Resetting flag after processing the section
}
// If not within the specified section, adding the number to the sum
if (inSection == 0)
{
sum += nums[i];
}
}
return sum; // Returning the calculated sum
}
int main()
{
int nums1[] = { 1, 5, 9, 10, 17 }; // Initializing array nums1 with various integers
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Displaying a message about the sum excluding specified numbers
cout << "Sum of the numbers of the said array except those numbers starting with 5 followed by at least one 6: " << endl;
// Calling test function with nums1 and displaying the resulting sum
cout << test(nums1, arr_length) << endl;
int nums2[] = { 1, 5, 6, 9, 10, 17 }; // Initializing array nums2 with various integers
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Displaying a message about the sum excluding specified numbers
cout << "Sum of the numbers of the said array except those numbers starting with 5 followed by at least one 6: " << endl;
// Calling test function with nums2 and displaying the resulting sum
cout << test(nums2, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 42 Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 37
Visual Presentation:
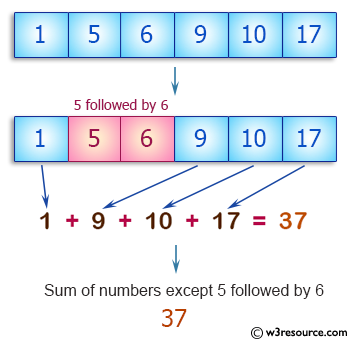
Flowchart:
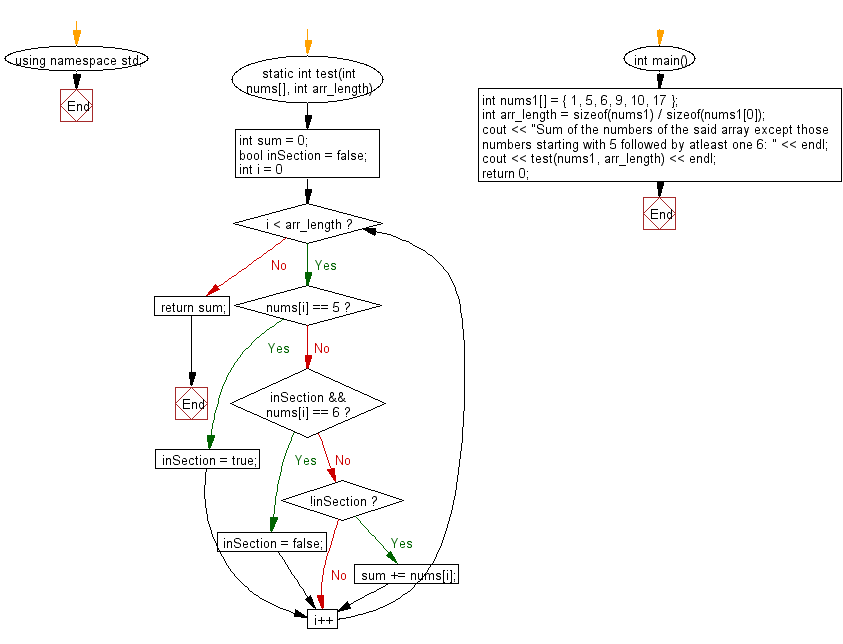
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
Next: Write a C++ program to check if a given array of integers contains 5 next to a 5 somewhere.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics