C++ Exercises: Check if a given positive number is a multiple of 3 or a multiple of 7
Multiple of 3 or 7
Write a C++ program to check if a given positive number is a multiple of 3 or a multiple of 7.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the given number is divisible by 3 or 7
bool test(int n)
{
return n % 3 == 0 || n % 7 == 0; // Returns true if the number is divisible by 3 or 7; otherwise, returns false
}
// Main function
int main()
{
cout << test(3) << endl; // Output the result of test function with number 3
cout << test(14) << endl; // Output the result of test function with number 14
cout << test(12) << endl; // Output the result of test function with number 12
cout << test(37) << endl; // Output the result of test function with number 37
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
1 1 1 0
Visual Presentation:
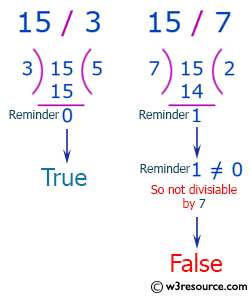
Flowchart:
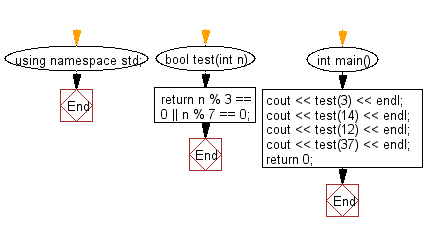
For more Practice: Solve these Related Problems:
- Write a C++ program to check if an input positive number is divisible by 3 or by 7, and print a boolean result.
- Write a C++ program that determines whether a given number is a multiple of 3 or a multiple of 7 using the modulo operator.
- Write a C++ program that reads a number and outputs true if it is divisible by 3 or 7, otherwise false.
- Write a C++ program to verify if a number is a multiple of either 3 or 7, ensuring proper input validation for positivity.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to create a new string with the last char added at the front and back of a given string of length 1 or more.
Next: Write a C++ program to create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back. If the given string length is less than 3, use whatever characters are there.
What is the difficulty level of this exercise?