C++ Exercises: Sort a given unsorted array of integers, in wave form
Write a C++ program to sort a given unsorted array of integers, in wave form.
Note: An array is in wave form when array[0] >= array[1] <= array[2] >= array[3] <= array[4] >= . . . .
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
#include<algorithm> // Header file for using algorithms like sort
using namespace std; // Using the standard namespace
void swap_elements(int *a, int *b)
{
int t = *a; // Temporary variable to store the value at pointer a
*a = *b; // Assigning the value at pointer b to pointer a
*b = t; // Assigning the value in the temporary variable to pointer b
}
void array_wave(int nums[], int n)
{
sort(nums, nums+n); // Sorting the array in ascending order using the sort function
// Loop to create the wave pattern in the array
for (int i = 0; i < n - 1; i += 2)
swap_elements(&nums[i], &nums[i+1]); // Swapping adjacent elements to create the wave pattern
}
int main()
{
int nums[] = {4, 5, 9, 12, 9, 22, 45, 7}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculating the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Outputting each element of the array
array_wave(nums, n); // Calling the function to create a wave pattern in the array
cout << "\nWave form of the said array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Outputting each element of the modified array in a wave pattern
return 0;
}
Sample Output:
Original array: 4 5 9 12 9 22 45 7 Wave form of the said array: 5 4 9 7 12 9 45 22
Flowchart:
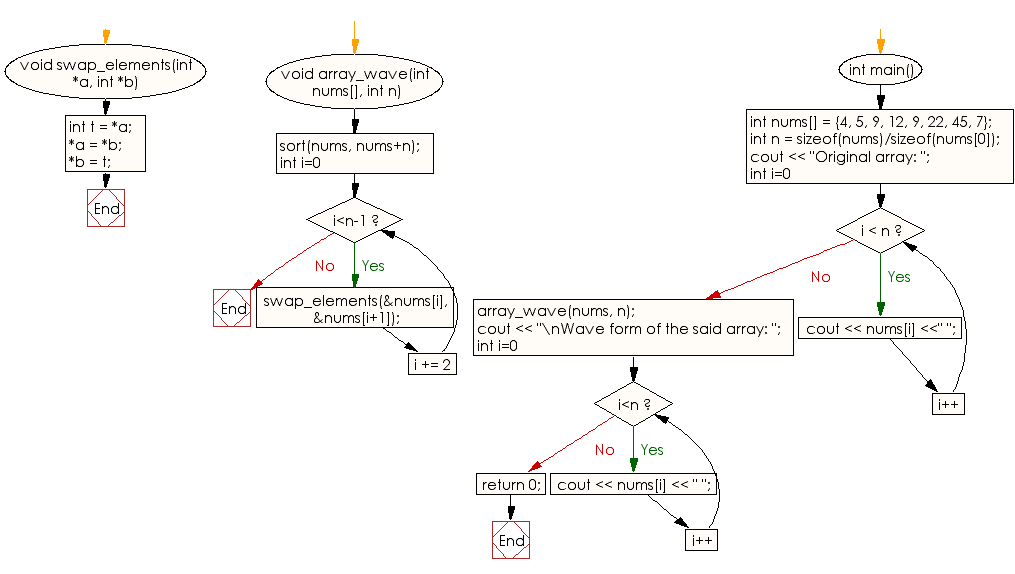
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the next greater element of every element of a given array of integers. Ignore those elements which have no greater element.
Next: Write a C++ program to find the smallest element missing in a sorted array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics