C++ Exercises: Find the next greater element of every element of a given array of integers
8. Next Greater Element for Every Array Element
Write a C++ program to find the next more powerful element of every element of a given array of integers. Ignore those elements that have no greater element.
Visual Presentation:
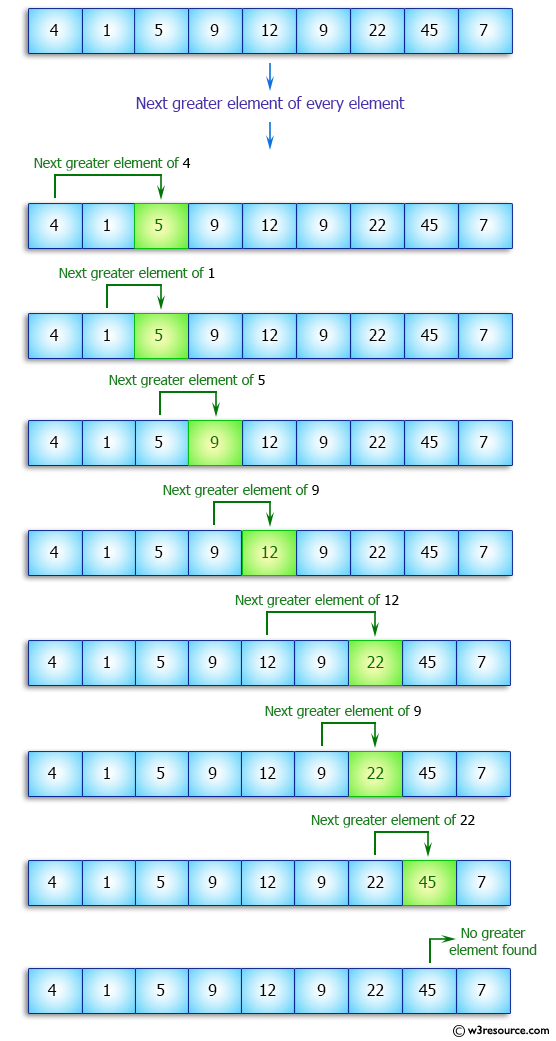
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
#include <stack> // Header file for using stack data structure
using namespace std; // Using the standard namespace
void next_greater(int nums[], int n)
{
stack<int> data_stack; // Declaring a stack to store elements
data_stack.push(nums[0]); // Pushing the first element of the array onto the stack
// Loop to find the next greater elements
for (int i = 1; i < n; i++)
{
int next_element = nums[i]; // Storing the current element to be compared
// Checking if the stack is not empty
if (data_stack.empty() == false)
{
int array_element = data_stack.top(); // Fetching the top element of the stack
data_stack.pop(); // Popping the top element from the stack
// Loop to find and print the next greater elements
while (array_element < next_element)
{
cout << array_element << ": " << next_element << endl; // Outputting the pair of elements
// Checking if the stack is empty
if (data_stack.empty() == true)
break;
array_element = data_stack.top(); // Fetching the top element of the stack
data_stack.pop(); // Popping the top element from the stack
}
// Checking if the current array element is greater than the next element
if (array_element > next_element)
data_stack.push(array_element); // Pushing the array element back onto the stack
}
data_stack.push(next_element); // Pushing the next element onto the stack
}
}
int main()
{
int nums[] = {4, 1, 5, 9, 12, 9, 22, 45, 7}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculating the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Outputting each element of the array
cout << "\nNext Greater Element:\n"; // Output message indicating the display of the next greater elements
next_greater(nums, n); // Calling the function to find the next greater elements
}
Sample Output:
Original array: 4 1 5 9 12 9 22 45 7 Next Greater Element: 1: 5 4: 5 5: 9 9: 12 9: 22 12: 22 22: 45
Flowchart:
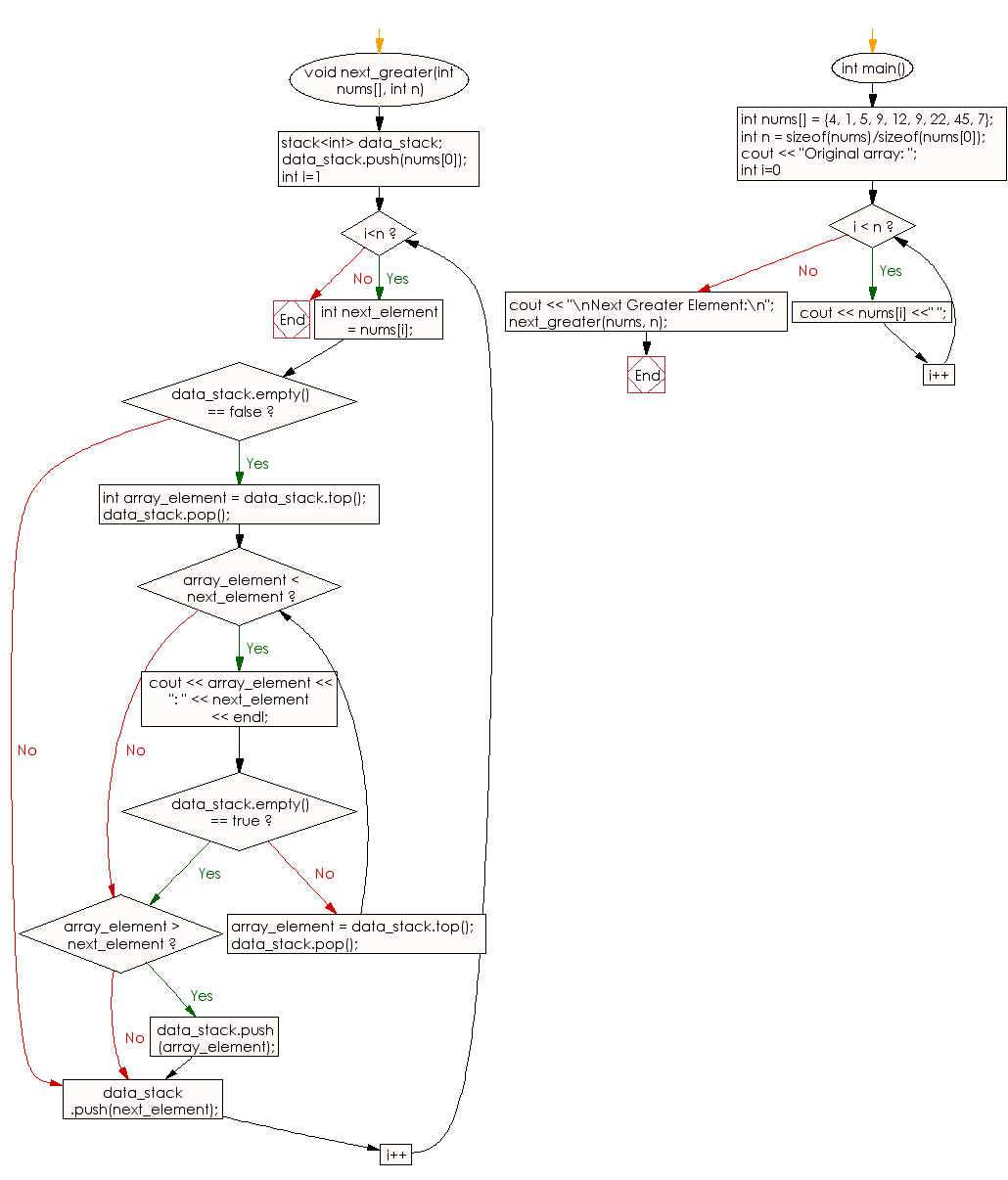
For more Practice: Solve these Related Problems:
- Write a C++ program to find the next greater element for each element in an array using a stack-based approach.
- Write a C++ program that iterates through an array and prints for each element the first element to its right that is greater, or -1 if none exists.
- Write a C++ program to determine and display the next greater element for every number in the array by using a single pass algorithm with a stack.
- Write a C++ program that reads an array and uses an auxiliary stack to compute the next higher element for each entry.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the most occurring element in an array of integers.
Next: Write a C++ program to sort a given unsorted array of integers, in wave form.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.