C++ Exercises: Find all elements in array of integers which have at-least two greater elements
6. Elements with At Least Two Significant Neighbors
Write a C++ program to find all elements in an array of integers that have at least two significant elements.
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to find elements in the array which have at least two greater elements
void find_greater_elements(int nums[], int n)
{
cout << "\nElements which have at-least two greater elements: "; // Output message to indicate displaying elements with at least two greater elements
for (int i=0; i<n; i++)
{
int ctr = 0; // Counter to track the number of elements greater than the current element
for (int j=0; j<n; j++)
if (nums[j] > nums[i]) // Checking if the element at index 'j' is greater than the element at index 'i'
ctr++; // Increment the counter if the condition is true
if (ctr >= 2) // Checking if the counter value is greater than or equal to 2
cout << nums[i] << " "; // Output the element which has at least two greater elements
}
}
int main()
{
int nums[] = {7, 12, 9, 15, 19, 32, 56, 70}; // Declaration and initialization of an integer array
int n = sizeof(nums)/sizeof(nums[0]); // Calculating the number of elements in the array
cout << "Original array: "; // Output message indicating the original array is being displayed
for (int i=0; i < n; i++)
cout << nums[i] <<" "; // Output each element of the array
find_greater_elements(nums, n); // Calling the function to find elements with at least two greater elements
return 0;
}
Sample Output:
Original array: 7 12 9 15 19 32 56 70 Elements which have at-least two greater elements: 7 12 9 15 19 32
Flowchart:
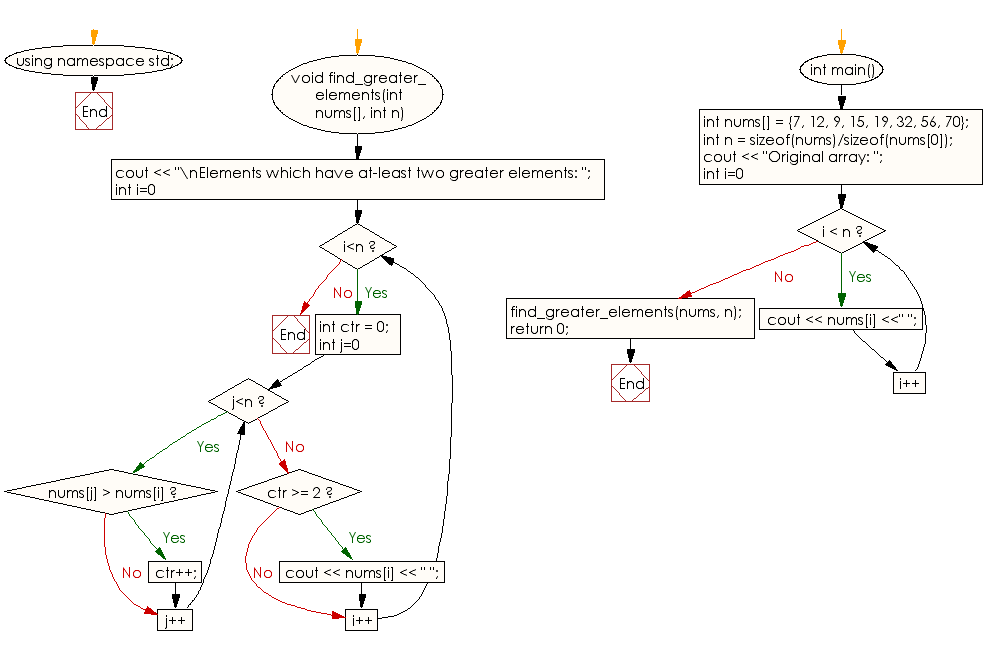
For more Practice: Solve these Related Problems:
- Write a C++ program to find all elements in an array that have at least two neighboring elements greater than a threshold value.
- Write a C++ program that reads an array and prints elements which are larger than both their immediate neighbors.
- Write a C++ program to output elements of an array that have at least two adjacent numbers satisfying a given condition.
- Write a C++ program to find and print all elements with two significant neighbors by comparing each element with the ones on its left and right.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the second smallest elements in a given array of integers.
Next: Write a C++ program to find the most occurring element in an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.