C++ Exercises: Find k largest elements in a given array of integers.
Write a C++ program to find the k largest elements in a given array of integers.
Visual Presentation:
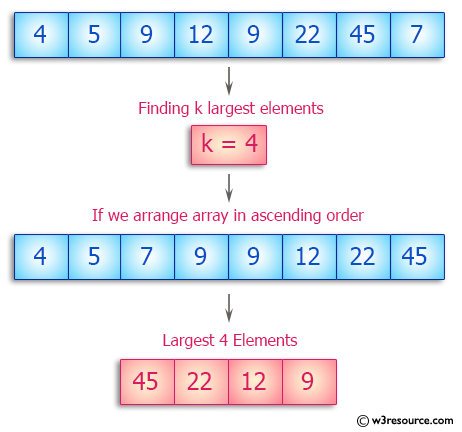
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to find and print the k largest elements in the array
void kLargest(int nums[], int n, int k)
{
sort(nums, nums+n, greater<int>()); // Sorting the array in descending order using sort function
cout << "\nLargest " << k << " Elements: "; // Output message indicating the k largest elements
for (int i = 0; i < k; i++) // Loop to print the k largest elements
cout << nums[i] << " "; // Output each of the k largest elements
}
int main() // Main function where the program execution starts
{
int nums[] = {4, 5, 9, 12, 9, 22, 45, 7}; // Declaration and initialization of an integer array
int n = sizeof(nums)/sizeof(nums[0]); // Determining the number of elements in the array
cout << "Original array: "; // Output message indicating the original array is being displayed
for (int i=0; i < n; i++)
cout << nums[i] <<" "; // Output each element of the array
int k = 4; // Number of largest elements to be displayed
kLargest(nums, n, k); // Calling function to find and print the k largest elements in the array
}
Sample Output:
Original array: 4 5 9 12 9 22 45 7 Largest 4 Elements: 45 22 12 9
Flowchart:
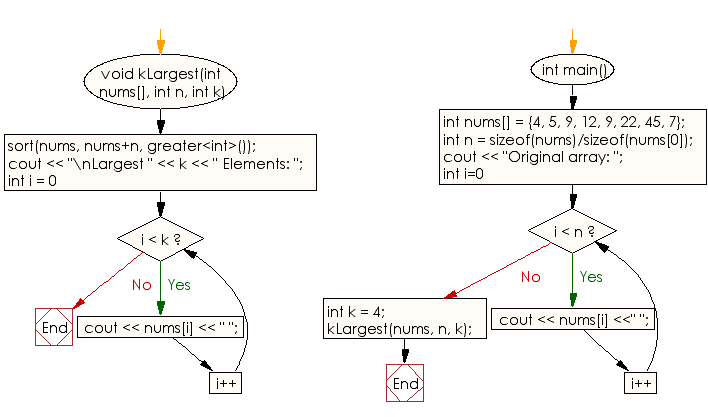
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find second largest element in a given array of integers.
Next: Write a C++ program to find the second smallest elements in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics