C++ Exercises: Find the number of pairs of integers in a given array of integers whose sum is equal to a specified number
27. Count Pairs with Sum Equal to Specified Number
Write a C++ program to find the number of pairs of integers in a given array of integers whose sum is equal to a specified number.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int main() // Main function
{
int array1[] = {1, 5, 7, 5, 8, 9, 11, 12}; // Declaration and initialization of the array
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of the array
cout << "Original array: "; // Output label for original array
for (int i = 0; i < s1; i++) // Loop to output elements of the original array
cout << array1[i] << " ";
int i, sum = 12, ctr = 0; // Declaration of variables for sum and count of pairs
cout << "\nArray pairs whose sum equal to 12: "; // Output label for pairs whose sum equals 12
for (int i = 0; i < s1; i++) // Loop through the array elements
{
for (int j = i + 1; j < s1; j++) // Nested loop to find pairs with sum equal to 12
{
if (array1[i] + array1[j] == sum) // Check if the sum of two elements is equal to 12
{
cout << "\n" << array1[i] << "," << array1[j]; // Output the pair
ctr++; // Increment count of pairs
}
}
}
cout << "\nNumber of pairs whose sum equals 12: "; // Output label for count of pairs
cout << ctr; // Output the count of pairs
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 1 5 7 5 8 9 11 12 Array pairs whose sum equal to 12: 1,11 5,7 7,5 Number of pairs whose sum equal to 12: 3
Flowchart:
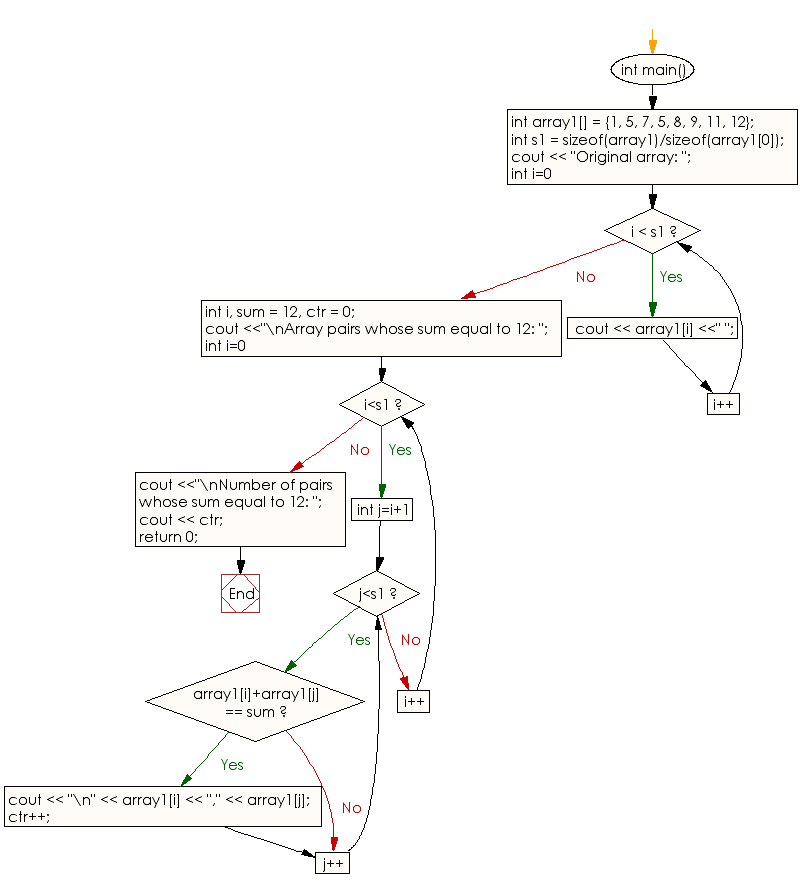
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of pairs in an array whose sum equals a given target using a nested loop.
- Write a C++ program that reads an array and a target sum, then uses a hash map to count all pairs that add up to the target.
- Write a C++ program to iterate over an array and count pair combinations whose sum matches a specified value, optimizing with two-pointer technique.
- Write a C++ program that sorts an array and then uses binary search to count the pairs with a sum equal to the given number.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find and print all unique elements of a given array of integers.
Next: Write a C++ program to arrange the numbers of a given array in a way that the sum of some numbers equal the largest number in the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.