C++ Exercises: Find and print all unique elements of a given array of integers
26. Distinct Elements in Array
Write a C++ program to find and print all distinct elements of a given array of integers.
Visual Presentation:
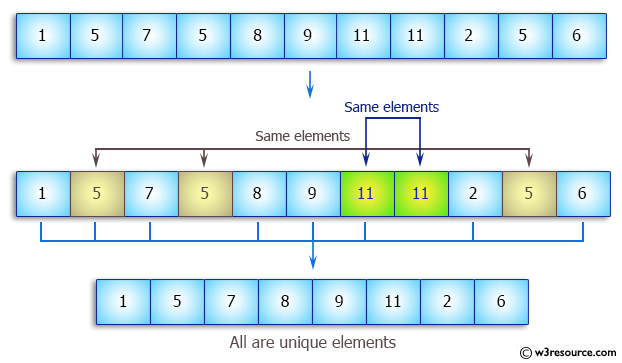
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int main() // Main function
{
int array1[] = {1, 5, 7, 5, 8, 9, 11, 11, 2, 5, 6}; // Declaration and initialization of the array
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of the array
cout << "Original array: "; // Output label for original array
for (int i = 0; i < s1; i++) // Loop to output elements of the original array
cout << array1[i] << " ";
cout << "\nUnique elements of the said array: "; // Output label for unique elements
for (int i = 0; i < s1; i++) // Loop to find and output unique elements
{
int j;
for (j = 0; j < i; j++) // Inner loop to check for duplicates until the current index i
{
if (array1[i] == array1[j]) // If a duplicate is found
break; // Break the loop
}
if (i == j) // If the index is equal to the inner loop's index j
cout << array1[i] << " "; // Output the unique element at index i
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 1 5 7 5 8 9 11 11 2 5 6 Unique elements of the said array: 1 5 7 8 9 11 2 6
Flowchart:
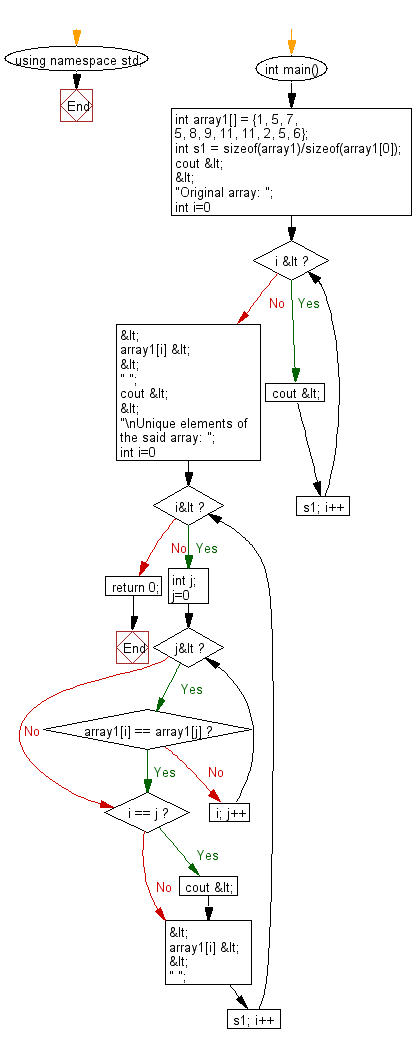
For more Practice: Solve these Related Problems:
- Write a C++ program to extract and print all distinct elements from an array using a set data structure.
- Write a C++ program that reads an array and outputs only the unique numbers by filtering duplicates.
- Write a C++ program to process an array and display distinct elements by using sorting and comparing adjacent values.
- Write a C++ program that iterates over an array and prints each element only once, even if it appears multiple times.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find and print all common elements in three sorted arrays of integers.
Next: Write a C++ program to find the number of pairs of integers in a given array of integers whose sum is equal to a specified number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.