C++ Exercises: Find and print all common elements in three sorted arrays of integers
25. Common Elements in Three Sorted Arrays
Write a C++ program to find and print all common elements in three sorted arrays of integers.
Visual Presentation:
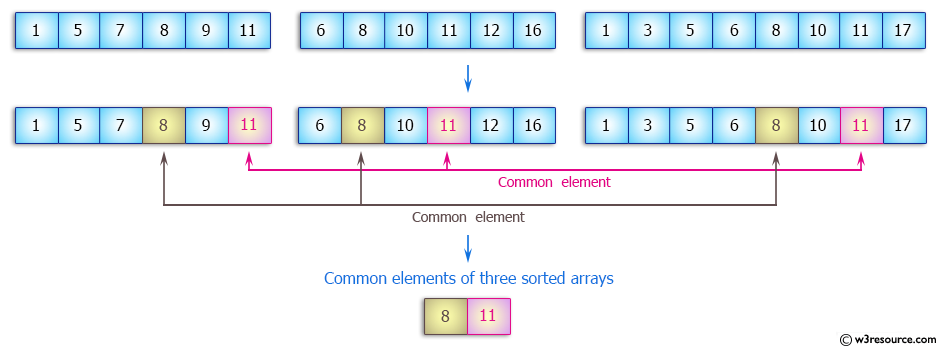
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int main() // Main function
{
int array1[] = {1, 5, 7, 8, 9, 11}; // Declaration and initialization of the first array
int array2[] = {6, 8, 10, 11, 12, 16}; // Declaration and initialization of the second array
int array3[] = {1, 3, 5, 6, 8, 10, 11, 17}; // Declaration and initialization of the third array
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of array1
int s2 = sizeof(array2) / sizeof(array2[0]); // Calculate the size of array2
int s3 = sizeof(array3) / sizeof(array3[0]); // Calculate the size of array3
cout << "Original arrays: "; // Output label for original arrays
cout << "\nArray1: ";
for (int i = 0; i < s1; i++) // Loop to output elements of array1
cout << array1[i] << " ";
cout << "\nArray2: ";
for (int i = 0; i < s2; i++) // Loop to output elements of array2
cout << array2[i] << " ";
cout << "\nArray3: ";
for (int i = 0; i < s3; i++) // Loop to output elements of array3
cout << array3[i] << " ";
cout << "\nCommon elements of the said sorted arrays: "; // Output label for common elements
int i = 0, j = 0, k = 0; // Initialize iterators for arrays
// Loop to find common elements among three sorted arrays
while (i < s1 && j < s2 && k < s3)
{
if (array1[i] == array2[j] && array2[j] == array3[k]) // If elements at current positions are equal
{
cout << array1[i] << " "; // Output the common element
i++;
j++;
k++;
}
else if (array1[i] < array2[j]) // If array1's element is less than array2's element
i++; // Move to the next element in array1
else if (array2[j] < array3[k]) // If array2's element is less than array3's element
j++; // Move to the next element in array2
else
k++; // Move to the next element in array3
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original arrays: Array1: 1 5 7 8 9 11 Array2: 6 8 10 11 12 16 Array3: 1 3 5 6 8 10 11 17 Common elements of the said sorted arrays: 8 11
Flowchart:
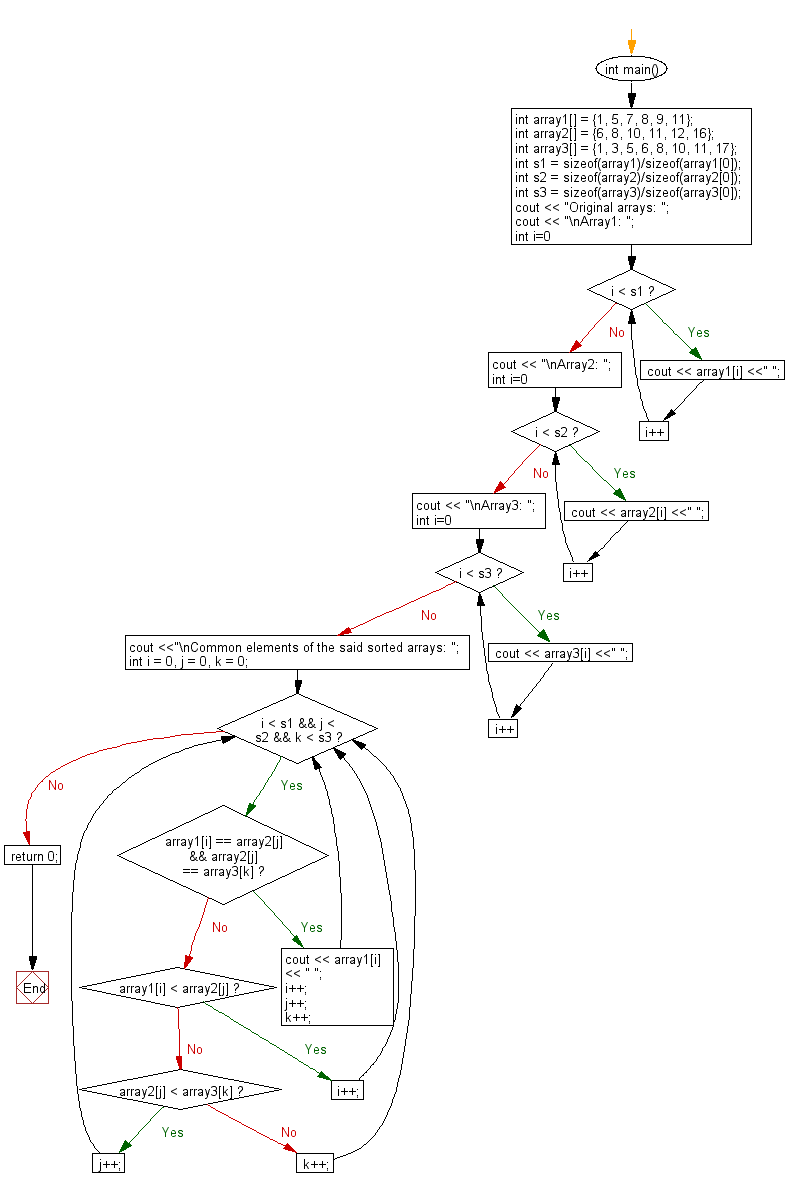
For more Practice: Solve these Related Problems:
- Write a C++ program to find and print all common elements in three sorted arrays using three pointers.
- Write a C++ program that reads three sorted arrays and outputs the intersection by advancing through them concurrently.
- Write a C++ program to compare three sorted arrays and display common numbers using a merge-like approach.
- Write a C++ program that utilizes the two-pointer technique extended to three arrays to find all shared elements.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the first repeating element in an array of integers.
Next: Write a C++ program to find and print all unique elements of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.