C++ Exercises: Find the first repeating element in an array of integers
24. Find the First Repeating Element in Array
Write a C++ program to find the first repeating element in an array of integers.
Visual Presentation:
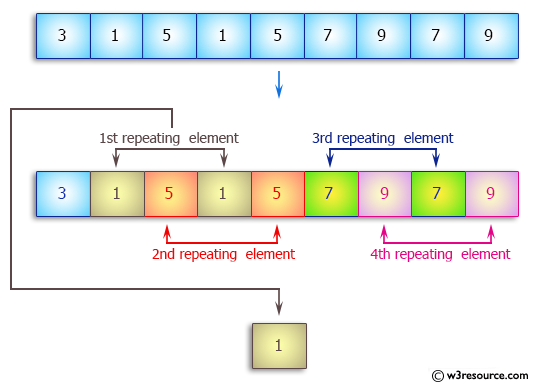
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
#include<set> // Header file for set container
using namespace std; // Using the standard namespace
// Function to find the first repeating element in an array
int first_repeating_element(int array1[], int s1)
{
int min_val = -1; // Initialize the minimum value as -1
set<int> result; // Create a set to store unique elements
// Traverse the array in reverse order
for (int i = s1 - 1; i >= 0; i--)
{
// If the element is already present in the set, update min_val
if (result.find(array1[i]) != result.end())
min_val = i;
else
result.insert(array1[i]); // Insert the element into the set
}
// Return the first repeating element found
if (min_val != -1)
return array1[min_val];
else
return 0;
}
// Main function
int main()
{
int array1[] = {3, 1, 5, 1, 5, 7, 9, 7, 9}; // Declaration and initialization of the array
int fre; // Variable to store the first repeating element
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of the array
cout << "Original array: "; // Output label for the original array
// Output each element of the original array
for (int i = 0; i < s1; i++)
cout << array1[i] << " ";
fre = first_repeating_element(array1, s1); // Call the function to find the first repeating element
cout << "\nFirst repeating element: " << fre; // Output the first repeating element
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 3 1 5 1 5 7 9 7 9 First repeating element: 1
Flowchart:
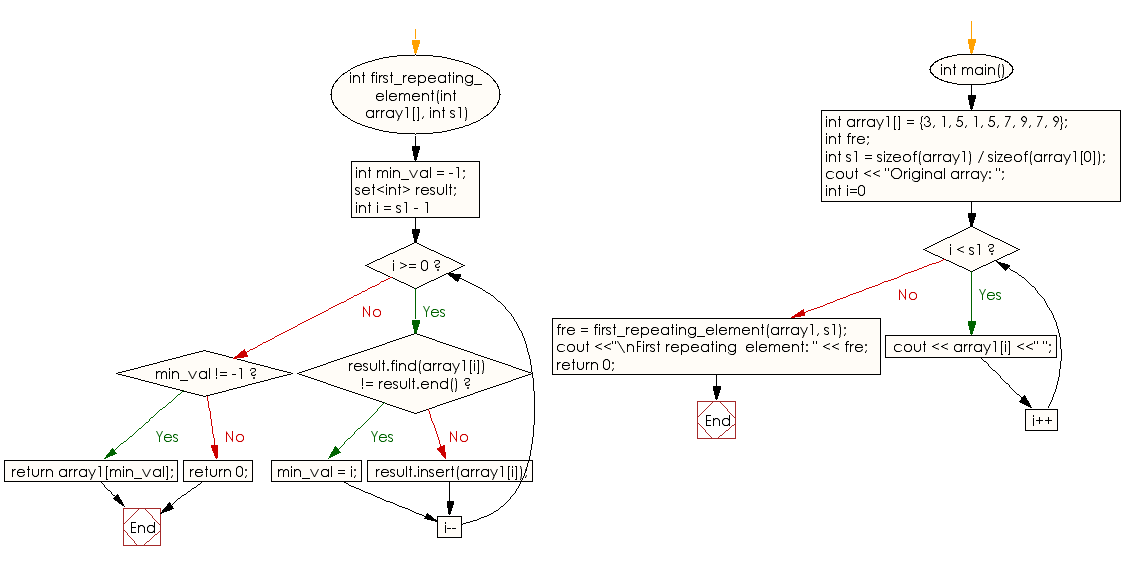
For more Practice: Solve these Related Problems:
- Write a C++ program to determine the first element in an array that repeats using a hash map for tracking indices.
- Write a C++ program that reads an array and prints the first repeating element by checking for duplicates in order.
- Write a C++ program to iterate over an array and return the first element whose count exceeds one.
- Write a C++ program to find the first duplicate element in an array by comparing each element with all previous elements.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the element that appears once in an array of integers and every other element appears twice.
Next: Write a C++ program to find and print all common elements in three sorted arrays of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.