C++ Exercises: Find the missing element from two given arrays of integers except one element
Write a C++ program to find the missing element from two given arrays of integers except one element.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int findMissing(int array1[], int array2[], int s1, int s2)
{
int result = 0; // Initialize a variable to store the missing number using bitwise XOR
for (int i = 0; i < s1; i++) // Loop through all elements of array1
result = result ^ array1[i]; // Perform bitwise XOR operation to find the missing number
for (int i = 0; i < s2; i++) // Loop through all elements of array2
result = result ^ array2[i]; // Perform bitwise XOR operation to find the missing number
return result; // Return the missing number found
}
int main()
{
int array1[] = {3, 1, 5, 7, 9}; // Declaration and initialization of the first array
int array2[] = {3, 7, 5, 9}; // Declaration and initialization of the second array
int mn; // Variable to store the missing number
int s1 = sizeof(array1) / sizeof(array1[0]); // Calculate the size of array1
int s2 = sizeof(array2) / sizeof(array2[0]); // Calculate the size of array2
// Check if the difference between the sizes of arrays is not more than 1
if (s1 != s2 - 1 && s2 != s1 - 1)
{
cout << "Invalid Input"; // Print an error message for invalid input
return 0; // Exit the program
}
cout << "Original arrays: "; // Output label for the original arrays
cout << "\nFirst array: "; // Output label for the first array
for (int i = 0; i < s1; i++)
cout << array1[i] << " "; // Output each element of the first array
cout << "\nSecond array: "; // Output label for the second array
for (int i = 0; i < s2; i++)
cout << array2[i] << " "; // Output each element of the second array
mn = findMissing(array1, array2, s1, s2); // Call the function to find the missing number
cout << "\nMissing number: " << mn; // Output the missing number
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original arrays: First array: 3 1 5 7 9 Second array: 3 7 5 9 Missing number: 1
Flowchart:
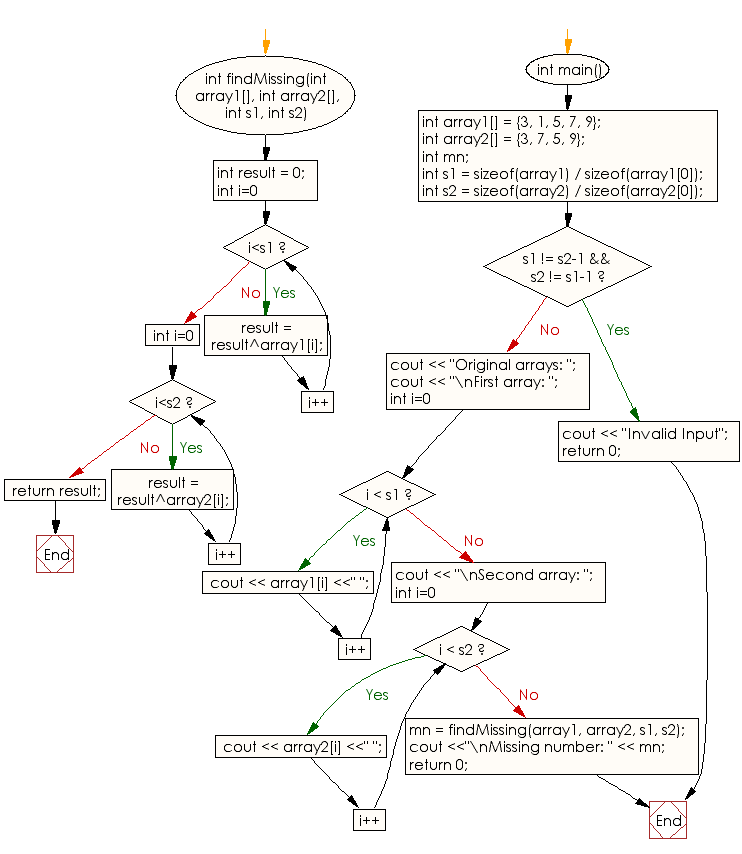
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the two repeating elements in a given array of integers.
Next: Write a C++ program to find the element that appears once in an array of integers and every other element appears twice.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics