C++ Exercises: Find the two repeating elements in a given array of integers
21. Find Two Repeating Elements in Array
Write a C++ program to find the two repeating elements in a given array of integers.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
int main()
{
int nums[] = {1, 2, 3, 5, 5, 7, 8, 8, 9, 9, 2}; // Declaration and initialization of an integer array
int i, j; // Declaration of loop control variables
int size = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (i = 0; i < size; i++)
cout << nums[i] << " "; // Output each element of the original array
cout << "\nRepeating elements: "; // Output label for repeating elements
for (i = 0; i < size; i++) // Outer loop to iterate through each element in the array
for (j = i + 1; j < size; j++) // Inner loop to compare each element with subsequent elements
if (nums[i] == nums[j]) // Check if current element is equal to any other element in the array
cout << nums[i] << " "; // Output the repeating element
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Original array: 1 2 3 5 5 7 8 8 9 9 2 Repeating elements: 2 5 8 9
Flowchart:
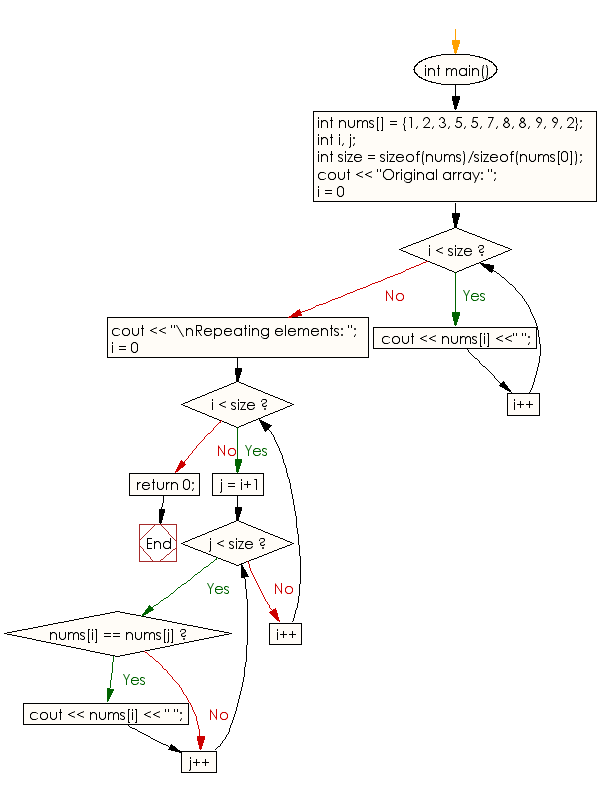
For more Practice: Solve these Related Problems:
- Write a C++ program to identify the two repeating elements in an array using a hash map to track frequencies.
- Write a C++ program that sorts the array and then finds the two elements that occur more than once by scanning for duplicates.
- Write a C++ program to detect and print the two duplicate elements in an array using nested loops with break conditions.
- Write a C++ program that reads an array and uses the sum and product properties to determine the two repeating numbers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count the number of occurrences of given number in a sorted array of integers.
Next: Write a C++ program to find the missing element from two given arrays of integers except one element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.