C++ Exercises: Sort a given array of 0s, 1s and 2s
Write a C++ program to sort a given array of 0s, 1s and 2s. In the final array put all 0s first, then all 1s and all 2s last.
Visual Presentation:
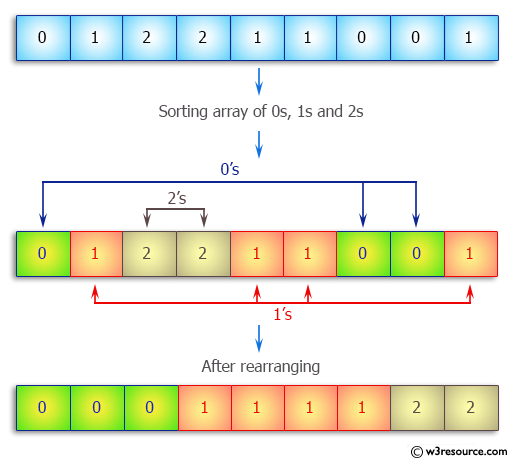
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to swap two integer values using pointers
void swap(int *x, int *y)
{
int temp = *x;
*x = *y;
*y = temp;
}
// Function to sort an array containing 0s, 1s, and 2s in ascending order
void sort_012_num(int nums[], int n)
{
int i = 0; // Pointer for 0s (left boundary)
int j = n - 1; // Pointer for 2s (right boundary)
int mid_num = 0; // Pointer for traversing the array
// Loop to arrange the elements
while (mid_num <= j)
{
switch (nums[mid_num])
{
case 0:
swap(&nums[i++], &nums[mid_num++]); // If the current element is 0, swap it with the left boundary element (0)
break;
case 1:
mid_num++; // If the current element is 1, move to the next element (no swap needed)
break;
case 2:
swap(&nums[mid_num], &nums[j--]); // If the current element is 2, swap it with the right boundary element (2)
break;
}
}
}
// Main function
int main()
{
int nums[] = {0, 1, 2, 2, 1, 1, 0, 0, 1}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
sort_012_num(nums, n); // Sort the array containing 0s, 1s, and 2s
cout << "\nArray elements after rearranging: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 2 2 1 1 0 0 1 Array elements after rearranging: 0 0 0 1 1 1 1 2 2
Flowchart:
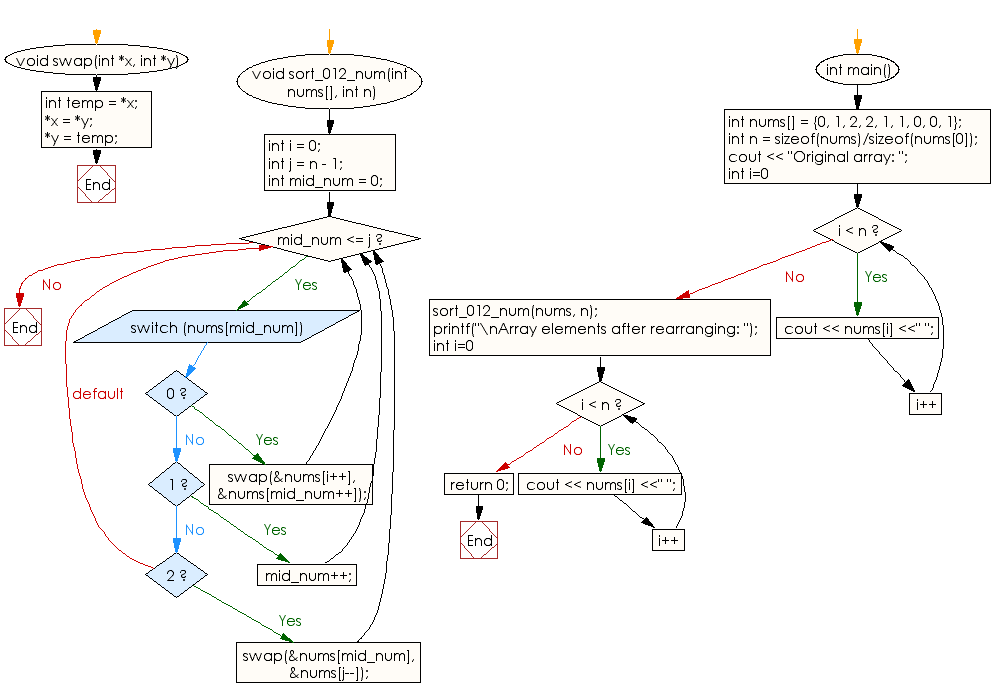
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to rearrange a given sorted array of positive integers.
Next: Write a C++ program to sort (in descending order) an array in of distinct elements according to absolute difference of array elements and with a given value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics