C++ Exercises: Rearrange a given sorted array of positive integers
15. Rearrangement of Sorted Array in Max-Min Order
Write a C++ program to rearrange a given sorted array of positive integers.
Note: In final array, first element should be maximum value, second minimum value, third second maximum value, fourth second minimum value, fifth third maximum and so on.
Sample Solution:
C++ Code :
#include <iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to rearrange elements such that first element is the maximum value, second is minimum value, third is second maximum, and so on.
void rearrange_max_min(int nums[], int n)
{
int temp[n]; // Temporary array to store rearranged elements
int small_num = 0, large_num = n - 1; // Initialize variables to track positions of smallest and largest elements
int result = true; // Variable to decide whether to pick maximum or minimum element
// Loop to rearrange elements in the temporary array
for (int i = 0; i < n; i++)
{
if (result)
temp[i] = nums[large_num--]; // Place the largest element at even index positions
else
temp[i] = nums[small_num++]; // Place the smallest element at odd index positions
result = !result; // Flip the result to switch between maximum and minimum elements
}
// Copy rearranged elements back to the original array
for (int i = 0; i < n; i++)
nums[i] = temp[i];
}
// Main function
int main()
{
int nums[] = {0, 1, 3, 4, 5, 6, 7, 8, 10}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
rearrange_max_min(nums, n); // Rearrange the array as per the requirement
cout << "\nArray elements after rearranging: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 3 4 5 6 7 8 10 Array elements after rearranging: 10 0 8 1 7 3 6 4 5
Flowchart:
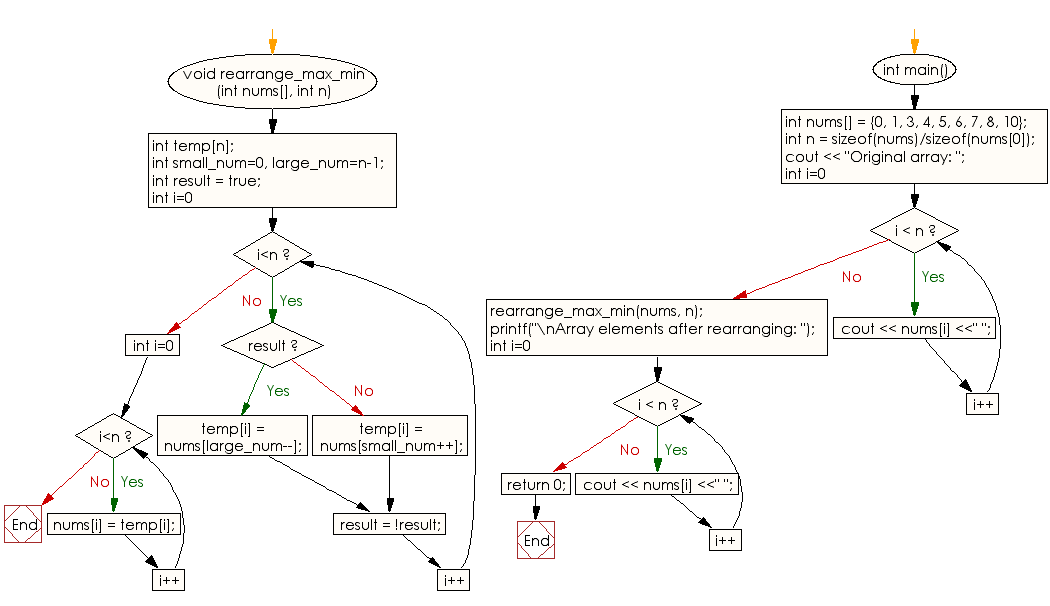
For more Practice: Solve these Related Problems:
- Write a C++ program to rearrange a sorted array so that the first element is the maximum, the second is the minimum, and so on.
- Write a C++ program that reads a sorted array and outputs a new array where elements alternate between the highest and lowest remaining values.
- Write a C++ program to interleave the maximum and minimum elements of a sorted array using two pointers from both ends.
- Write a C++ program that processes a sorted array and prints it in a max-min alternating order without extra memory allocation.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to separate 0s and 1s from a given array of values 0 and 1.
Next: Write a C++ program to sort a given array of 0s, 1s and 2s. In the final array put all 0s first, then all 1s and all 2s in last.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.