C++ Exercises: Separate 0s and 1s from a given array of values 0 and 1
14. Separate 0s and 1s from an Array
Write a C++ program to separate 0s and 1s from a given array of values 0 and 1.
Visual Presentation:
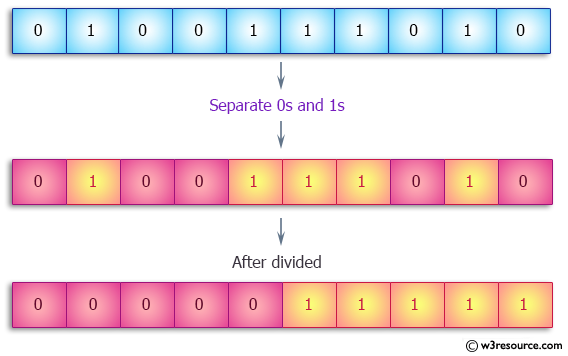
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to segregate 0s and 1s in the array
void segregateEvenOdd(int nums[], int n)
{
int ctr = 0; // Initialize a counter for the number of zeroes
// Count the number of zeroes in the array
for (int i = 0; i < n; i++) {
if (nums[i] == 0)
ctr++;
}
// Place the counted zeroes at the beginning of the array
for (int i = 0; i < ctr; i++)
nums[i] = 0;
// Place the remaining ones after the zeroes in the array
for (int i = ctr; i < n; i++)
nums[i] = 1;
}
// Main function
int main()
{
int nums[] = {0, 1, 0, 0 , 1, 1, 1, 0, 1, 0}; // Declaration and initialization of an integer array
int n = sizeof(nums)/sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i=0; i < n; i++)
cout << nums[i] <<" "; // Output each element of the original array
segregateEvenOdd(nums, n); // Rearrange the array by segregating 0s and 1s
cout << "\nArray after segregation: ";
for (int i=0; i < n; i++)
cout << nums[i] <<" "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 0 0 1 1 1 0 1 0 Array after divided: 0 0 0 0 0 1 1 1 1 1
Flowchart:
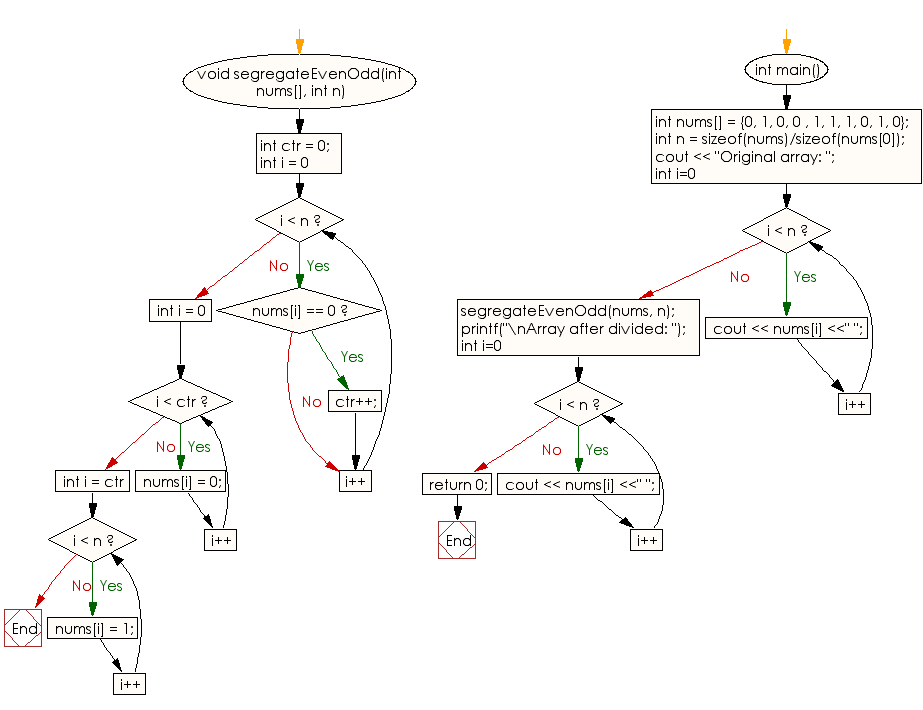
For more Practice: Solve these Related Problems:
- Write a C++ program to rearrange an array containing only 0s and 1s so that all 0s appear before all 1s.
- Write a C++ program that reads an array of binary values and partitions it into two sections, with 0s first.
- Write a C++ program to sort an array of 0s and 1s using a two-pointer approach without extra space.
- Write a C++ program that uses counting sort to rearrange an array consisting of only 0s and 1s.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to separate even and odd numbers of an array of integers. Put all even numbers first, and then odd numbers.
Next: Write a C++ program to rearrange a given sorted array of positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.